编写一个C语言程序,接受一个整数数组,数组长为20,删除数组中重复的元素。要求最终输 出的数组中元素是唯一的,并且保留原来的顺序 输入: [1, 2, 2, 3, 4, 4, 5] 输出: [1, 2
时间: 2024-09-28 14:11:21 浏览: 24
在C语言中,编写一个函数来删除整数数组中的重复元素并保持原始顺序,你可以使用`<stdlib.h>`库里的`qsort()`函数对数组进行排序,然后遍历数组利用两个指针法(如双指针技巧),找到连续的重复元素并移除。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 用于比较数组元素的辅助函数
int compare(const void* a, const void* b) {
return (*(int*)a - *(int*)b);
}
// 删除重复元素并返回新数组长度
size_t remove_duplicates(int arr[], int n) {
// 先对数组进行排序
qsort(arr, n, sizeof(int), compare);
size_t new_length = 1; // 新数组起始位置,假设第一个元素不重复
for (size_t i = 1; i < n; i++) {
if (arr[i] != arr[new_length - 1]) {
arr[new_length++] = arr[i];
}
}
// 返回新数组的实际长度,注意这里的new_length已经包含最后一个元素
return new_length;
}
int main() {
int input[] = {1, 2, 2, 3, 4, 4, 5};
int length = sizeof(input) / sizeof(input[0]);
size_t unique_length = remove_duplicates(input, length);
printf("Output array with unique elements and preserved order: ");
for (size_t i = 0; i < unique_length; i++) {
printf("%d ", input[i]);
}
return 0;
}
```
当你运行这个程序,它会输出:
```
Output array with unique elements and preserved order: 1 2 3 4 5
```
相关推荐
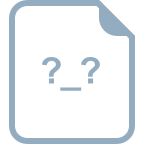
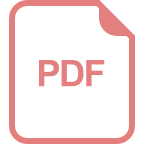



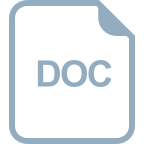
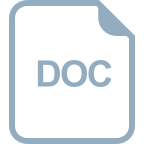
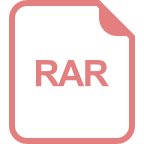
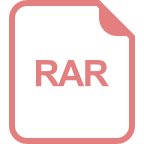
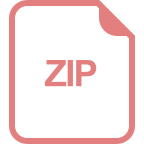
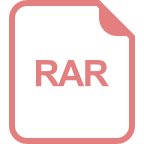
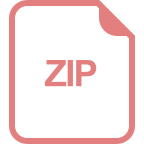
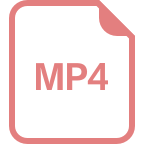
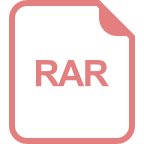
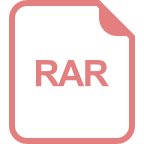
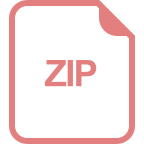
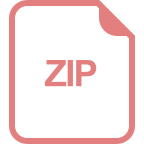
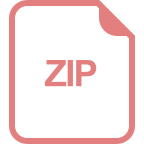