python字典pop方法
时间: 2024-08-11 19:01:53 浏览: 102
在Python中,`pop()`是一个内置方法,用于从字典(dict)中删除并返回指定键对应的值。如果键存在,则返回该键的值,然后移除这个键值对;如果键不存在,你可以提供一个默认值作为参数,程序会返回这个默认值而不是抛出KeyError异常。其基本语法如下:
```python
value = dict.pop(key[, default])
```
- `key`: 需要删除并返回其值的键。
- `default` (可选): 如果`key`不存在,返回此默认值,默认为`None`。
例如:
```python
my_dict = {'a': 1, 'b': 2}
# 删除并返回'a'对应的值
value = my_dict.pop('a')
print(value) # 输出:1
print(my_dict) # 输出:{'b': 2}
# 如果尝试删除不存在的键,可以设置默认值
value_not_found = my_dict.pop('c', 'Default Value')
print(value_not_found) # 输出:'Default Value'
```
相关问题
python字典pop
在Python中,`pop()` 是一个内置方法,用于从字典中删除并返回指定键对应的值。如果提供了键,该方法将从字典中移除该键值对,并返回对应的值;如果键不存在,你可以选择提供一个默认值(当键不存在时返回的值),或者引发`KeyError`异常。
基本语法如下:
```python
dict.pop(key, default=None)
```
- `key`: 需要移除的键
- `default`: 可选,默认为`None`。如果`key`不存在,返回这个默认值,否则返回被删除的值
相关问题--
1. 字典的`pop()`方法有没有默认行为?
2. 如果尝试删除一个不存在的键,会有什么后果?
3. 在什么情况下会用到`default`参数?
python字典操作方法
Python中对字典进行操作的方法有以下几种:
1. 使用del关键字删除字典中的特定项。例如,使用del dictionary[key]可以删除字典中的某个键值对。
2. 使用clear()方法清空整个字典。调用dictionary.clear()会将字典中的所有项都删除,使其变为空字典。
3. 使用pop(key)方法删除字典中指定键的项,并返回该项的值。例如,使用dictionary.pop(key)可以删除字典中指定键的项,并返回该项的值。
4. 使用popitem()方法随机删除字典中一个键值对,并返回被删除的键值对。这个方法在Python 3.7之前是随机选择最后一个键值对进行删除,在Python 3.7及之后的版本中,它删除的是字典中的任意一个键值对。
以上是Python中对字典进行常用操作的方法。字典是一种无序的对象集合,通过键来存取元素。可以使用不可变对象作为键,最常用的类型是字符串和元组。字典在数据处理中非常实用,可以快速访问和操作数据。
阅读全文
相关推荐
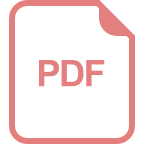
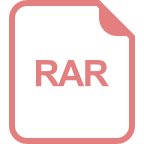
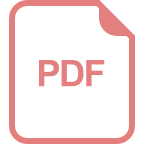
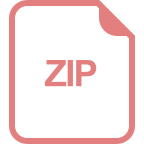
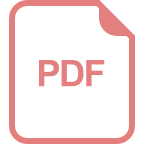
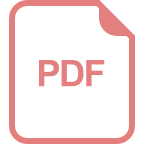
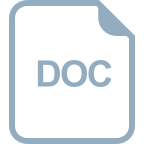
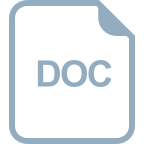








