qt QCustomPlot 动态添加曲线
时间: 2024-12-27 17:22:22 浏览: 22
### 使用 Qt 和 QCustomPlot 动态添加曲线
为了在 Qt 中使用 QCustomPlot 动态添加曲线,可以按照以下方式编写代码。这涉及到初始化 `QCustomPlot` 小部件、设置图形属性以及定期向图表中添加新的数据点。
#### 初始化和配置 QCustomPlot
首先,在项目的 `.pro` 文件中加入对 QCustomPlot 的支持:
```makefile
QT += core gui widgets
CONFIG += c++17
SOURCES += main.cpp \
mainwindow.cpp
HEADERS += mainwindow.h
RESOURCES += resources.qrc
FORMS += mainwindow.ui
LIBS += -L/path/to/qcustomplot/lib -lqcustomplot
INCLUDEPATH += /path/to/qcustomplot/include
```
接着,在主窗口类中声明并实例化 `QCustomPlot` 对象,并对其进行基本配置:
```cpp
// mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include "ui_mainwindow.h"
#include "qcustomplot.h"
class MainWindow : public QMainWindow {
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
private slots:
void updateGraph();
};
#endif // MAINWINDOW_H
// mainwindow.cpp
#include "mainwindow.h"
#include <QTimer>
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 创建一个新的 QCustomPlot 实例
QCustomPlot* customPlot = new QCustomPlot(this);
setCentralWidget(customPlot);
// 设置坐标轴标签
customPlot->xAxis->setLabel("Time");
customPlot->yAxis->setLabel("Value");
// 启用自动缩放功能以便适应新增加的数据点
customPlot->rescaleAxes();
// 开启定时器每隔一秒触发一次更新函数
QTimer* timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, &MainWindow::updateGraph);
timer->start(1000); // 每隔一秒钟调用一次 updateGraph 函数
}
void MainWindow::updateGraph() {
static int timeIndex = 0;
QVector<double> xData{timeIndex}, yData{(double)(rand())/(RAND_MAX/10)};
// 获取当前的第一个图层 (graph),如果没有则创建它
if (!customPlot->graphCount())
customPlot->addGraph();
// 更新现有图层上的 X/Y 数据集
customPlot->graph()->setData(xData, yData);
// 增量增加时间索引
++timeIndex;
// 自动调整坐标范围来显示最新添加的数据点
customPlot->xAxis->setRange(timeIndex-9, timeIndex+1);
customPlot->replot();
}
```
上述代码展示了如何通过继承自 `QWidget` 或其他基类的窗体控件集成 `QCustomPlot` 并实现简单的动态绘图逻辑[^4]。
阅读全文
相关推荐




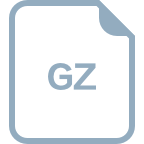













