vue3+vite+element-plus实现全部全选
时间: 2024-10-17 22:12:36 浏览: 71
在 Vue3 中结合 Vite 和 Element Plus 实现表格的全选功能,通常你会创建一个复选框或者单击列头的按钮来控制所有行的状态。以下是一个简化的步骤:
1. **安装依赖**:
首先确保已安装 Vue、Vite 和 Element Plus。如果尚未安装,可以分别运行以下命令:
```
npm install -g create-vite
npx create-vite your-project-name
cd your-project-name
npm install element-plus vue3 vite-plugin-element-plus
```
2. **引入组件**:
在 `src/components` 目录下创建一个 `Table.vue` 文件,并导入 Element 的表格 (`<el-table>`), 全选框 (`<el-checkbox-group>`) 和分页组件 (`<el-pagination>`), 如果有需要。
3. **数据结构和方法**:
在组件中,维护一个数组状态来存储表格的所有选中项,比如 `selectedItems`。定义一个方法如 `toggleAllSelection` 来切换全选状态。
```html
<template>
<div>
<el-table :data="tableData" :key="item.id">
<!-- ... -->
<el-column type="selection" width="55"></el-column>
<!-- ... -->
<el-row slot-scope="scope" @click="handleRowClick(scope.$index)">
<!-- ... -->
</el-row>
</el-table>
<el-checkbox-group v-model="selectedItems">
<el-checkbox v-for="item in tableData" :label="item.id" :key="item.id"></el-checkbox>
</el-checkbox-group>
<el-pagination />
</div>
</template>
<script setup>
import { ref } from 'vue';
import { useTable } from 'element-plus';
const selectedItems = ref([]);
const tableData = ref([]); // 这里假设你已经有了 tableData 数据
// toggleAllSelection 方法
function toggleAllSelection(isChecked) {
if (isChecked) {
selectedItems.value = tableData.value.map(item => item.id);
} else {
selectedItems.value = [];
}
}
// 使用 Element Plus 表格插件并处理点击事件
const table = useTable({
data,
rowKey: 'id',
methods: {
handleRowClick(index) {
const isChecked = !selectedItems.value.includes(tableData.value[index].id);
toggleAllSelection(isChecked);
},
},
});
</script>
```
4. **使用全选按钮**:
如果你想添加一个单独的全选按钮,可以在模板中添加一个按钮并绑定到 `toggleAllSelection` 函数。
现在你应该已经实现了表格的全选功能。你可以通过点击复选框或者全选按钮来选择或取消选择所有行。
阅读全文
相关推荐
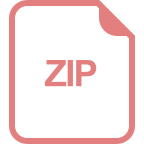
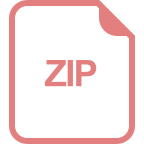
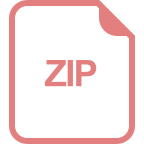
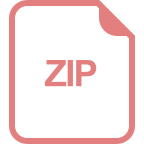
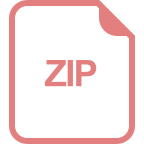
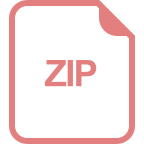
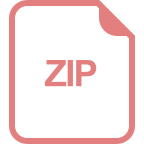
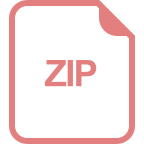
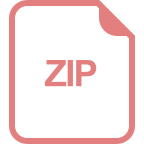
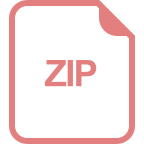
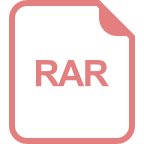
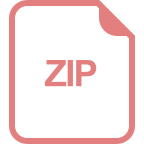
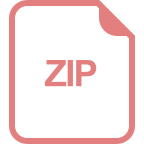
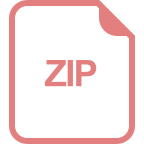
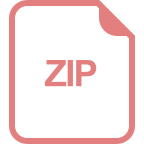
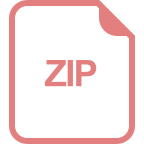
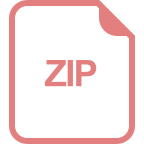
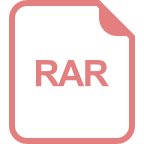