WINFORM做一个功能,在搜索页面可以搜索出档案列表,列表包含档案路径,需要实现的功能是,点击档案路径,直接弹出共享文件夹,该文件夹设置为只读的账号,且不用每次打开文件夹都需要输入账号密码,请写出完整代码
时间: 2024-09-09 08:11:02 浏览: 49
在WinForm中,实现这个功能需要用到`WebBrowser`控件来展示文件夹内容,因为`WebBrowser`可以直接嵌入HTML并支持JavaScript。下面是一个简单的示例代码,假设已知的共享文件夹URL是`http://your-server/path/to/shared/folder`,并且使用预先设置好的只读账户。
```csharp
using System.Windows.Forms;
using System.Net.Http;
using System.Text.RegularExpressions;
public partial class MainForm : Form
{
private WebBrowser browser;
public MainForm()
{
InitializeComponent();
browser = new WebBrowser();
Controls.Add(browser);
browser.Dock = DockStyle.Fill;
browser.ScrollBarsEnabled = false;
// 创建一个匿名的HttpClient实例,用于获取HTML内容
HttpClient client = new HttpClient();
string htmlContent = await client.GetStringAsync("http://your-server/path/to/shared/folder");
SetFolderContent(htmlContent);
}
private void SetFolderContent(string html)
{
// 解析HTML,找到档案链接,将它们替换为点击后跳转到只读共享文件夹的链接
Regex regex = new Regex(@"<a href='(.+?)'>"); // 根据实际HTML结构调整正则表达式
MatchCollection matches = regex.Matches(html);
foreach (Match match in matches)
{
string link = match.Groups[1].Value;
string updatedLink = $"file:///your-read-only-folder?path={Uri.EscapeDataString(link)}";
html = html.Replace(link, updatedLink);
}
browser.DocumentText = html;
// 注册JavaScript事件处理函数,当点击档案路径时打开共享文件夹
browser.Document.InvokeScript("addEventHandler", new object[] { "a", "onclick", GetOpenFolderFunction });
}
private JavaScriptCallbackResult GetOpenFolderFunction(object sender, ElementClickEventArgs e)
{
// 获取当前点击链接的path参数
var path = new Uri(e.Element.DomElement.href).QueryParameters["path"];
if (path != null)
{
// 打开只读文件夹
Process.Start($"file:///your-read-only-folder?path={path}");
}
return null;
}
}
// 注意:这个例子中的"your-server"、"your-read-only-folder"以及正则表达式都需要根据实际情况调整。
```
在这个例子中,`GetOpenFolderFunction`会被浏览器自动执行,当用户点击档案链接时,它会启动一个新的进程打开指定的只读文件夹。请注意,实际项目中应该考虑使用更安全的方式来传递路径信息,例如使用POST请求,而不是硬编码在URL中。
**相关问题--**:
1. 如何确保只读账户的安全?
2. 如果服务器地址和文件夹路径发生变化如何更新代码?
3. 如何处理点击无效链接或者网络连接问题?
阅读全文
相关推荐
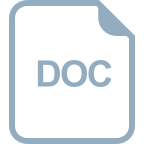
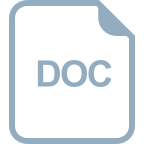
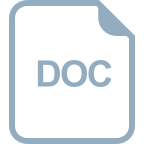
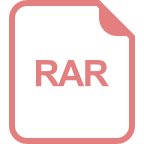
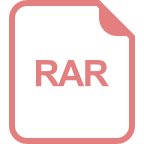
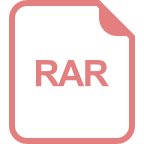
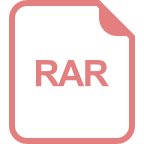
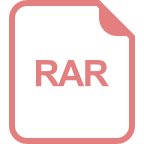
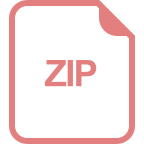
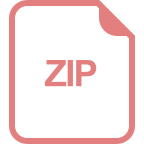
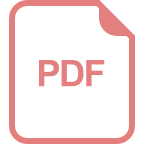
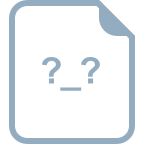
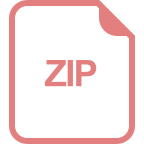
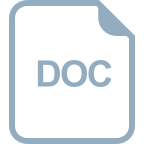
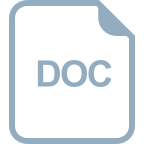
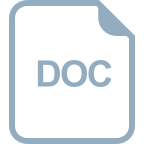
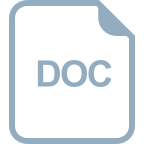
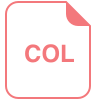
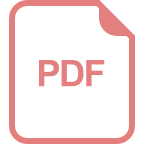