linux中frambuffer写一个显示BMP格式图片的完整程序
时间: 2024-04-14 07:26:10 浏览: 20
下面是一个完整的Linux下使用帧缓冲显示BMP格式图片的程序:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <linux/fb.h>
#include <sys/mman.h>
#include <sys/ioctl.h>
void drawBMP(const char *filename) {
int fbfd = open("/dev/fb0", O_RDWR);
if (fbfd == -1) {
perror("Error: cannot open framebuffer device");
return;
}
struct fb_var_screeninfo vinfo;
if (ioctl(fbfd, FBIOGET_VSCREENINFO, &vinfo) == -1) {
perror("Error: reading variable screen info");
close(fbfd);
return;
}
int screensize = vinfo.xres * vinfo.yres * vinfo.bits_per_pixel / 8;
char *fbp = (char *)mmap(0, screensize, PROT_READ | PROT_WRITE, MAP_SHARED, fbfd, 0);
if ((int)fbp == -1) {
perror("Error: failed to map framebuffer device to memory");
close(fbfd);
return;
}
FILE *bmpfile = fopen(filename, "rb");
if (bmpfile == NULL) {
perror("Error: cannot open BMP file");
munmap(fbp, screensize);
close(fbfd);
return;
}
unsigned char bmpheader[54];
if (fread(bmpheader, sizeof(unsigned char), 54, bmpfile) != 54) {
perror("Error: invalid BMP file");
fclose(bmpfile);
munmap(fbp, screensize);
close(fbfd);
return;
}
int width = *(int *)&bmpheader[18];
int height = *(int *)&bmpheader[22];
int bpp = *(int *)&bmpheader[28];
if (bpp != 24) {
perror("Error: BMP file must be 24 bits per pixel");
fclose(bmpfile);
munmap(fbp, screensize);
close(fbfd);
return;
}
unsigned char *bmpdata = (unsigned char *)malloc(screensize);
if (fread(bmpdata, sizeof(unsigned char), screensize, bmpfile) != screensize) {
perror("Error: invalid BMP file");
fclose(bmpfile);
free(bmpdata);
munmap(fbp, screensize);
close(fbfd);
return;
}
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
long location = (x + vinfo.xoffset) * (vinfo.bits_per_pixel / 8) +
(y + vinfo.yoffset) * vinfo.bytes_per_line;
unsigned char b = bmpdata[(x + (width * (height - y - 1))) * 3];
unsigned char g = bmpdata[(x + (width * (height - y - 1))) * 3 + 1];
unsigned char r = bmpdata[(x + (width * (height - y - 1))) * 3 + 2];
unsigned int color = ((r << vinfo.red.offset) & vinfo.red.msb) |
((g << vinfo.green.offset) & vinfo.green.msb) |
((b << vinfo.blue.offset) & vinfo.blue.msb);
*((unsigned int *)(fbp + location)) = color;
}
}
fclose(bmpfile);
free(bmpdata);
munmap(fbp, screensize);
close(fbfd);
}
int main() {
drawBMP("image.bmp");
return 0;
}
```
请注意,您需要将要显示的BMP图片命名为"image.bmp"并与程序文件放在同一目录下。编译并运行该程序,它将会使用帧缓冲显示图片。请确保在运行程序时具有适当的权限(通常需要root权限)。
相关推荐







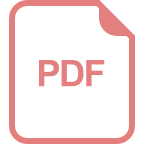
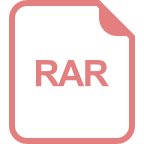
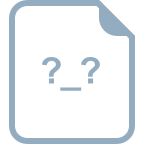
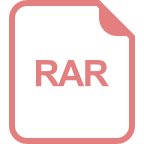
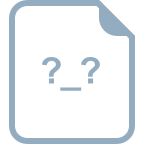