python读取csv的方法
时间: 2023-11-21 11:58:16 浏览: 95
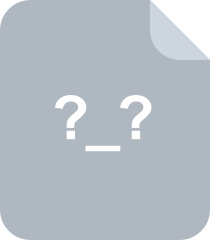
python读取csv文件方法
以下是两种Python读取CSV文件的方法:
1. 使用codecs.open()和csv.DictReader()方法:
```python
import codecs
import csv
with codecs.open('file.csv', encoding='utf-8-sig') as f:
for row in csv.DictReader(f, skipinitialspace=True):
print(row)
```
2. 使用open()和csv.reader()方法:
```python
import csv
with open('file.csv', encoding='utf-8-sig') as f:
for row in csv.reader(f, skipinitialspace=True):
print(row)
```
其中,第一种方法使用了codecs库来打开文件并指定编码方式,然后使用csv.DictReader()方法读取CSV文件并返回一个字典类型的迭代器,可以通过遍历迭代器来逐行读取CSV文件的内容。
第二种方法直接使用open()方法打开文件,然后使用csv.reader()方法读取CSV文件并返回一个列表类型的迭代器,同样可以通过遍历迭代器来逐行读取CSV文件的内容。
阅读全文
相关推荐
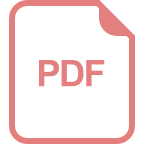
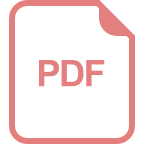
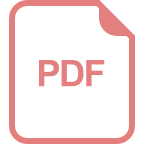









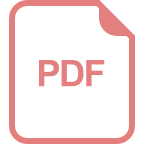
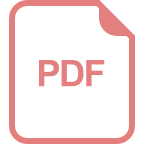
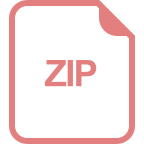