如何在Java中实现滑动窗口限流算法以控制请求速率?请提供详细的代码实现和性能分析。
时间: 2024-10-30 09:18:09 浏览: 21
在处理高并发系统时,滑动窗口限流算法是一种有效的方法来控制请求速率,保证系统的稳定性。它能够将时间窗口均匀划分为多个小的时间段,每个时间段内都有独立的请求计数,从而实现对流量的精细控制。
参考资源链接:[Java实现限流策略:计数器与滑动窗口](https://wenku.csdn.net/doc/3ihvf301fi?spm=1055.2569.3001.10343)
在Java中实现滑动窗口限流,我们可以采用数组或队列来记录每个时间段内的请求计数。以下是一个基本的滑动窗口算法实现的示例代码,使用数组作为数据结构来存储每个时间段的计数器,并提供了一个检查请求是否通过限流的方法:
```java
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.ConcurrentHashMap;
public class SlidingWindowLimiter {
private final int capacity;
private final long windowSize;
private final long period;
private final ConcurrentHashMap<Long, AtomicInteger> timestamps;
private final ConcurrentHashMap<Long, AtomicInteger> counts;
public SlidingWindowLimiter(int capacity, long windowSize, long period) {
this.capacity = capacity;
this.windowSize = windowSize;
this.period = period;
this.timestamps = new ConcurrentHashMap<>();
this.counts = new ConcurrentHashMap<>();
}
public boolean tryAcquire(long timestamp) {
long currentWindowStart = timestamp - (timestamp % period);
timestamps.put(currentWindowStart, new AtomicInteger(1));
counts.put(currentWindowStart, new AtomicInteger(1));
// Remove timestamps and counts that are outside the current window.
timestamps.entrySet().removeIf(entry -> entry.getKey() < currentWindowStart - windowSize);
counts.entrySet().removeIf(entry -> entry.getKey() < currentWindowStart - windowSize);
return timestamps.values().stream()
.mapToInt(AtomicInteger::get)
.sum() <= capacity;
}
}
```
在上述代码中,我们使用了`ConcurrentHashMap`来存储每个时间窗口的开始时间和对应的计数器,以确保线程安全。当新请求到来时,我们检查当前时间窗口内的请求计数是否超过设定的限流容量。如果请求计数未超过容量,则允许该请求通过,并更新计数器。同时,我们会定期清理已经过期的时间窗口数据,以避免内存溢出。
为了提高性能,可以对上述基础实现进行优化,例如使用固定大小的数组代替`ConcurrentHashMap`来减少内存分配和锁竞争,或者引入分段锁技术来减少锁的粒度。
当选择滑动窗口算法时,需要考虑算法的内存和计算开销,尤其是在时间窗口划分较为细致的情况下。在实际应用中,还可以根据具体的业务场景和性能要求,调整窗口大小和容量限制,以达到最佳的限流效果。
关于滑动窗口限流算法的更多细节和深入分析,推荐阅读《Java实现限流策略:计数器与滑动窗口》。该资料详细介绍了滑动窗口算法的原理及其在Java中的实现,有助于理解算法的性能影响并选择合适的实现策略。
参考资源链接:[Java实现限流策略:计数器与滑动窗口](https://wenku.csdn.net/doc/3ihvf301fi?spm=1055.2569.3001.10343)
阅读全文
相关推荐
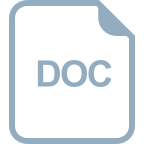
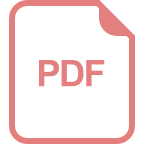
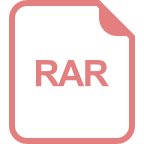
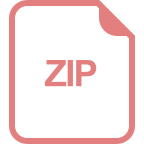
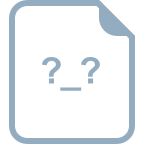
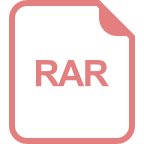
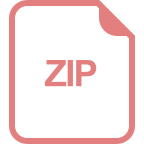
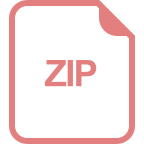
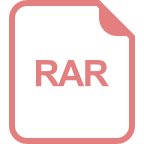
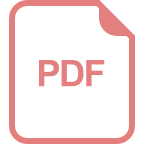
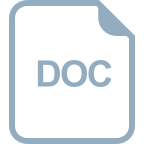
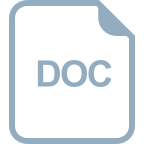
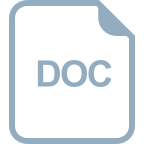
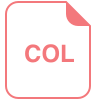
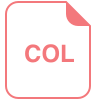


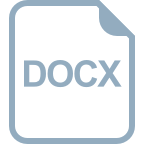