我有一个vue页面的表格,该表格已经实现了新建、编辑和删除功能,新建会弹出一个表单需要用户输入文件名称和上传文件。这个表格有两列,分别是文件名称列和操作列,操作列有两个按钮分别是预览和下载,现在我需要你帮我实现点击预览按钮就能打开已经上传过的对应文件而不下载,点击下载按钮就直接下载文件而不会打开文件
时间: 2024-02-03 12:12:29 浏览: 14
对于预览功能,可以通过以下步骤实现:
1. 在表格中添加一个列来显示预览按钮,并为每个按钮设置一个唯一的标识符,比如文件ID。
2. 当用户点击预览按钮时,将该文件ID作为参数发送到后端API,并获得该文件的URL。
3. 将该URL用window.open()方法打开,以在新窗口中显示文件。
代码示例:
```
<template>
<el-table :data="tableData">
<el-table-column prop="fileName" label="文件名称"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button type="text" @click="previewFile(scope.row.id)">预览</el-button>
<el-button type="text" @click="downloadFile(scope.row.id)">下载</el-button>
</template>
</el-table-column>
</el-table>
</template>
<script>
export default {
data() {
return {
tableData: [
{ id: 1, fileName: '文件1' },
{ id: 2, fileName: '文件2' }
]
}
},
methods: {
previewFile(id) {
// 发送文件ID到后端API获取文件URL
const fileUrl = getFileUrl(id);
// 打开文件URL以预览文件
window.open(fileUrl, '_blank');
},
downloadFile(id) {
// 发送文件ID到后端API获取文件URL
const fileUrl = getFileUrl(id);
// 创建a标签并模拟点击以下载文件
const link = document.createElement('a');
link.href = fileUrl;
link.download = true;
link.click();
}
}
}
</script>
```
对于下载功能,可以通过以下步骤实现:
1. 在表格中添加一个列来显示下载按钮,并为每个按钮设置一个唯一的标识符,比如文件ID。
2. 当用户点击下载按钮时,将该文件ID作为参数发送到后端API,并获得该文件的URL。
3. 创建一个a标签并设置href属性为该文件URL,并设置download属性为true。
4. 触发a标签的click事件以模拟用户点击下载链接。
代码示例:
```
<template>
<el-table :data="tableData">
<el-table-column prop="fileName" label="文件名称"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button type="text" @click="previewFile(scope.row.id)">预览</el-button>
<el-button type="text" @click="downloadFile(scope.row.id)">下载</el-button>
</template>
</el-table-column>
</el-table>
</template>
<script>
export default {
data() {
return {
tableData: [
{ id: 1, fileName: '文件1' },
{ id: 2, fileName: '文件2' }
]
}
},
methods: {
previewFile(id) {
// 发送文件ID到后端API获取文件URL
const fileUrl = getFileUrl(id);
// 打开文件URL以预览文件
window.open(fileUrl, '_blank');
},
downloadFile(id) {
// 发送文件ID到后端API获取文件URL
const fileUrl = getFileUrl(id);
// 创建a标签并模拟点击以下载文件
const link = document.createElement('a');
link.href = fileUrl;
link.download = true;
link.click();
}
}
}
</script>
```
相关推荐
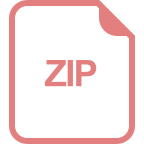














