.net framework封装udp
时间: 2023-09-29 21:06:16 浏览: 46
在 .NET Framework 中,可以使用 System.Net.Sockets 命名空间中的 UdpClient 类来封装 UDP(用户数据报协议)。
以下是一个简单的示例,演示如何在 .NET Framework 中使用 UdpClient 类封装 UDP:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
class Program
{
static void Main(string[] args)
{
// 创建 UdpClient 对象,并指定要监听的本地端口号
UdpClient udpClient = new UdpClient(1234);
try
{
// 接收数据
IPEndPoint remoteEP = null;
byte[] data = udpClient.Receive(ref remoteEP);
// 处理接收到的数据
Console.WriteLine("Received data from {0}:", remoteEP.ToString());
Console.WriteLine(Encoding.ASCII.GetString(data));
// 发送响应数据
string responseData = "Response data";
byte[] responseBytes = Encoding.ASCII.GetBytes(responseData);
udpClient.Send(responseBytes, responseBytes.Length, remoteEP);
Console.WriteLine("Sent response data to {0}:", remoteEP.ToString());
}
catch (SocketException e)
{
Console.WriteLine("SocketException: {0}", e);
}
finally
{
// 关闭 UdpClient 对象
udpClient.Close();
}
}
}
```
在上面的示例中,我们创建了一个 UdpClient 对象,并指定要监听的本地端口号。然后,我们调用 UdpClient.Receive 方法来接收数据。该方法将在数据到达时阻塞,直到接收到数据为止。接收到数据后,我们可以处理它们,然后使用 UdpClient.Send 方法发送响应数据。
需要注意的是,UdpClient 类是可重用的,可以多次使用。在使用完 UdpClient 对象后,需要调用其 Close 方法来关闭它。
相关推荐
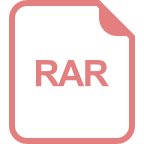
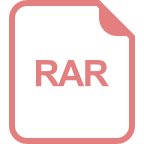
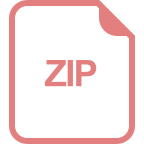














