使用C语言设计一个程序,该程序能够从文件中读取小学整数加减乘除的测试数据和相应的运算符号,然后让用户输入计算结果,根据答案判断并输出’太棒了,你答对了!‘或’很遗憾,继续努力!’,同时确保程序能正确处理所有可能的输入情况
时间: 2024-10-25 12:08:42 浏览: 26
要设计一个C语言程序来完成这个任务,首先需要做的是定义结构体来存储输入的数据,包括数值和运算符。接着,编写函数读取文件,解析输入并执行计算。最后,比较用户的输入与预期答案,给出反馈。
以下是一个基本的框架:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义结构体存储数值和运算符
typedef struct {
int number;
char operator; // '+', '-', '*', '/'
} Operation;
// 函数用于从文件中读取数据
Operation read_operation_from_file(const char *filename) {
FILE *file = fopen(filename, "r");
if (!file) {
perror("Error opening file");
exit(EXIT_FAILURE);
}
Operation op;
fscanf(file, "%d %c", &op.number, &op.operator); // 假设文件格式为 'number operator'
fclose(file);
return op;
}
// 根据运算符执行计算
int calculate(int num1, char op, int num2) {
switch (op) {
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
if (num2 != 0)
return num1 / num2;
else
printf("Error: Division by zero.\n");
return -1; // 返回错误代码
default:
printf("Invalid operator.\n");
return -1; // 返回错误代码
}
}
// 主函数,接收用户输入并比较
int main() {
const char* filename = "test_data.txt"; // 假设文件名
Operation expected_op = read_operation_from_file(filename);
int user_input;
printf("Enter the result of %d %c %d: ", expected_op.number, expected_op.operator, expected_op.number);
if (scanf("%d", &user_input) == 1) { // 用户输入一个整数
int result = calculate(expected_op.number, expected_op.operator, expected_op.number);
if (result == -1) {
printf("Please try again.\n");
} else if (result == user_input) {
printf("太棒了, 你答对了!\n");
} else {
printf("很遗憾,继续努力!预期结果是%d。\n", result);
}
} else {
printf("Invalid input. Please enter an integer.\n");
}
return 0;
}
```
阅读全文
相关推荐
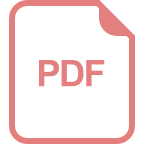
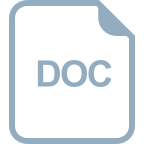
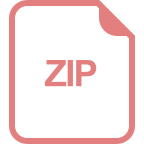

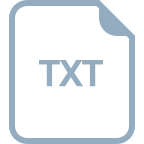
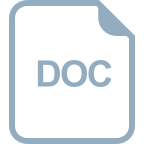
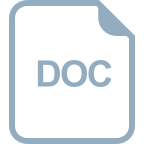
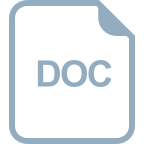
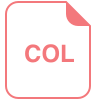
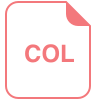
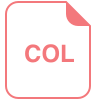
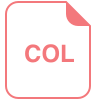
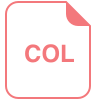
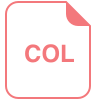
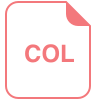
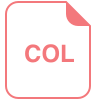

