Expected all tensors to be on the same device, but found at least two devices, cuda:0 and cpu!
时间: 2023-10-14 08:20:26 浏览: 20
这个错误提示表明你的代码中有两个或多个张量分别存储在不同的设备上,一个在 GPU 上,一个在 CPU 上。要解决这个问题,你需要将这些张量都移到同一设备上。可以使用`.to()`方法将张量转移到指定的设备上,例如:
```python
import torch
# 创建一个在 GPU 上的张量
x_gpu = torch.ones((3, 3)).cuda()
# 创建一个在 CPU 上的张量
x_cpu = torch.ones((3, 3))
# 将 x_cpu 转移到 GPU 上
x_cpu = x_cpu.to('cuda')
# 现在两个张量都在 GPU 上了
print(x_gpu.device) # 输出:cuda:0
print(x_cpu.device) # 输出:cuda:0
```
在你的代码中找到并修复这个问题,确保所有张量都在同一设备上即可。
相关问题
Expected all tensors to be on the same device, but found at least two devices, cuda:1 and cpu!
该错误通常出现在使用PyTorch进行深度学习模型训练时,其中一些张量在GPU上,另一些张量在CPU上。这可能是因为在将模型参数或数据加载到设备时出现了问题。
解决这个问题的一种方法是确保所有的张量都在同一个设备上。你可以使用以下步骤来解决问题:
1. 首先,确定你的GPU是否可用,可以使用以下代码来检查:
```
import torch
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
print(device)
```
2. 确保所有的模型参数和数据都在同一个设备上。你可以使用`.to(device)`方法将它们转移到所需的设备上。例如:
```
model.to(device)
data = data.to(device)
```
3. 如果你的模型中有自定义的网络层或函数,确保它们也在设备上。你可以在forward方法中使用`.to(device)`将它们转移到所需的设备上。
4. 如果仍然遇到问题,你可以尝试使用`.cpu()`方法将所有的张量转移到CPU上,然后再使用`.to(device)`将它们转移到所需的设备上。
总之,确保所有的张量和模型参数都在同一个设备上,并使用`.to(device)`将它们转移到所需的设备上,应该可以解决这个问题。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [解决【Expected all tensors to be on the same device, but found at least two devices,】](https://blog.csdn.net/renkke/article/details/125298679)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
- *3* [Expected all tensors to be on the same device, but found at least two devices, cuda:0 and cpu!](https://blog.csdn.net/qq_43138237/article/details/126003221)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
Expected all tensors to be on the same device, but found at least two devices, cuda:0 and cpu! (when
这个错误通常是由于在混合使用GPU和CPU时出现的。它表示在计算过程中,有两个或多个张量被分配到了不同的设备上,一个在GPU上,一个在CPU上。
为了解决这个问题,你可以使用`.to()`方法将所有的张量都移动到同一个设备上。例如,如果你想将所有的张量都移动到GPU上,可以使用以下代码:
```python
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# 将所有的张量移动到同一个设备上
tensor1 = tensor1.to(device)
tensor2 = tensor2.to(device)
...
```
这样就可以确保所有的张量都在同一个设备上进行计算,避免出现设备不匹配的错误。
相关推荐
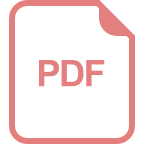
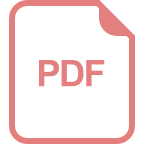







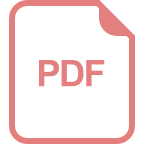
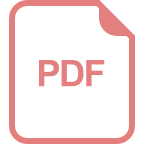
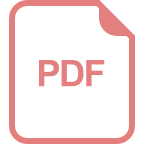
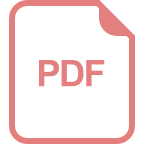
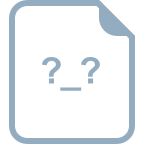