使用spring security框架实现权限以及登录,如何自定义密码校验
时间: 2023-01-11 22:55:36 浏览: 124
使用 Spring Security 框架实现权限和登录时,可以通过扩展 `AuthenticationProvider` 接口来自定义密码校验。
具体步骤如下:
1. 实现 `AuthenticationProvider` 接口
2. 在 `authenticate` 方法中实现密码校验的逻辑
3. 在 Spring Security 配置文件中配置自定义的 `AuthenticationProvider`
4. 在登录表单中使用自定义的密码校验逻辑进行登录
示例代码:
```java
import org.springframework.security.authentication.AuthenticationProvider;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.AuthenticationException;
public class CustomAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
String username = authentication.getName();
String password = authentication.getCredentials().toString();
// 使用自定义的密码校验逻辑进行校验
if (checkPassword(username, password)) {
return new UsernamePasswordAuthenticationToken(username, password, emptyList());
} else {
throw new BadCredentialsException("Invalid username or password");
}
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
```
```xml
<!-- 配置自定义的 AuthenticationProvider -->
<authentication-manager>
<authentication-provider ref="customAuthenticationProvider"/>
</authentication-manager>
```
阅读全文
相关推荐





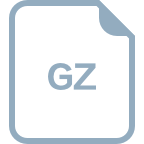









