// 输入通道 type Inlet interface { In() chan<- interface{} } // 输出通道 type Outlet interface { Out() <-chan interface{} } // Source步骤 type Source interface { Outlet // 设置回调接口 Via(Flow) Flow } // Flow步骤 type Flow interface { Inlet Outlet Via(Flow) Flow To(Target) } // Target步骤 type Target interface { Inlet // 确认操作完毕 } 使用 Connect将他们连接起来
时间: 2024-01-15 21:03:21 浏览: 120
假设我们有一个 `Source`、两个 `Flow` 和一个 `Target`,可以使用 `Connect` 函数将它们连接起来,代码如下:
```
type MySource struct {
outlet Outlet
}
func (s *MySource) Outlet() Outlet {
if s.outlet == nil {
s.outlet = make(chan interface{})
}
return s.outlet
}
func (s *MySource) Via(flow Flow) Flow {
flow.Inlet().In() <- <-s.Outlet().Out()
return flow
}
type MyFlow struct {
inlet Inlet
outlet Outlet
}
func (f *MyFlow) Inlet() Inlet {
if f.inlet == nil {
f.inlet = make(chan interface{})
}
return f.inlet
}
func (f *MyFlow) Outlet() Outlet {
if f.outlet == nil {
f.outlet = make(chan interface{})
}
return f.outlet
}
func (f *MyFlow) Via(flow Flow) Flow {
go func() {
for {
flow.Inlet().In() <- <-f.Outlet().Out()
}
}()
return flow
}
func (f *MyFlow) To(tgt Target) {
go func() {
for {
data := <-f.Outlet().Out()
tgt.Inlet().In() <- data
}
}()
}
type MyTarget struct {
inlet Inlet
}
func (t *MyTarget) Inlet() Inlet {
if t.inlet == nil {
t.inlet = make(chan interface{})
}
return t.inlet
}
func (t *MyTarget) Acknowledge(data interface{}) {
// do something with data
}
func main() {
src := &MySource{}
flow1 := &MyFlow{}
flow2 := &MyFlow{}
tgt := &MyTarget{}
Connect(src, []Flow{flow1, flow2}, tgt)
}
```
在上述代码中,我们定义了 `MySource`、`MyFlow` 和 `MyTarget` 三个结构体,并分别实现了 `Source`、`Flow` 和 `Target` 接口。在 `main` 函数中,我们创建了一个 `MySource` 对象、两个 `MyFlow` 对象和一个 `MyTarget` 对象,并调用 `Connect` 函数将它们连接起来。需要注意的是,这里我们使用了自定义的实现,实际项目中可能需要根据具体需求进行修改。
阅读全文
相关推荐
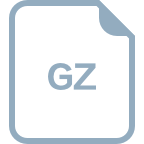
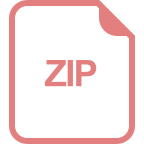




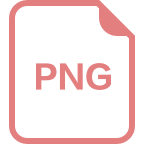
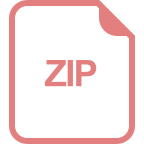
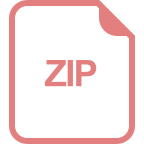
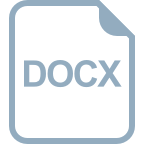
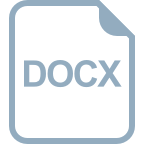