import logging def log_file(): log_file = 'testfun.log' handler_test = logging.FileHandler(log_file)
时间: 2024-05-04 18:21:21 浏览: 11
这段代码定义了一个名为 `log_file` 的函数,该函数用于初始化一个文件日志处理器,将日志输出到指定的文件中。具体来说,该函数中的代码:
- 创建了一个变量 `log_file`,并将其赋值为字符串 `'testfun.log'`,表示日志文件的名称为 `testfun.log`。
- 创建了一个名为 `handler_test` 的文件日志处理器,该处理器通过 `logging.FileHandler` 方法创建,并将日志输出到 `log_file` 所指定的文件中。
需要注意的是,由于该函数没有返回值,因此调用该函数并不会得到任何返回值。如果想要使用 `handler_test` 处理器来记录日志,需要在函数外部进行调用。例如,可以使用以下代码获取一个名为 `logger` 的日志对象,并将其绑定到 `handler_test` 处理器上,从而实现将日志输出到文件中:
```python
import logging
def log_file():
log_file = 'testfun.log'
handler_test = logging.FileHandler(log_file)
logger = logging.getLogger()
logger.addHandler(handler_test)
log_file()
logging.info('This message will be logged to testfun.log')
```
相关问题
logging.FileHandler(log_path)报错PermissionError: [Errno 13] Permission denied:
当您在尝试使用logging.FileHandler(log_path)创建一个日志文件时,可能会出现PermissionError: [Errno 13] Permission denied:这个错误。这通常是因为您的程序没有足够的权限来在指定的目录中创建文件。
为了解决这个问题,您可以尝试以下几种方法:
1. 以管理员身份运行程序:在Windows上,您可以右键单击程序图标并选择“以管理员身份运行”来提高程序的权限。
2. 更改目录权限:如果您没有管理员权限,则可以尝试更改目标目录的权限。右键单击目标目录并选择“属性”>“安全性”>“编辑”,然后添加您的用户名并授予“完全控制”权限。
3. 更改日志文件夹位置:如果您无法更改目标目录的权限,则可以将日志文件写入其他位置,例如您的用户主目录或者桌面。
希望这些方法可以帮助您解决这个问题。
from utils import get_module_version log = utils.setup_logging(__name__)
这段代码的作用是什么?能否解释一下其中的函数和变量?
```python
from utils import get_module_version
log = utils.setup_logging(__name__)
```
其中, `get_module_version` 是从 `utils` 模块中导入的一个函数,用于获取模块的版本信息。
`log` 是一个日志记录器,使用 `utils` 模块中的 `setup_logging` 函数进行设置。这个函数会返回一个日志记录器对象,我们可以通过这个对象来记录日志信息。
在这里,`__name__` 代表当前模块的名称,也就是说,`log` 日志记录器将记录当前模块中的日志信息。
总体来说,这段代码的作用是设置日志记录器,并导入一个用于获取模块版本信息的函数。这样,在代码中就可以方便地记录日志信息,并获取模块版本信息。
相关推荐
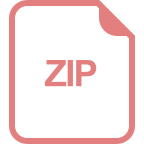
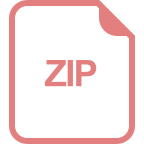












