【问题】使用python完成四则运算(用字典实现)。 【输入】在一行中输入一个数字 在一行中输入一个四则运算符(+,-,*,/) 在一行中输入一个数字。 【输出】运算结果(小数保留2位
时间: 2024-05-13 07:15:38 浏览: 167
以下是使用字典实现四则运算的Python代码:
```python
# 定义一个字典,将运算符和对应的函数进行关联
operators = {
'+': lambda x, y: x + y,
'-': lambda x, y: x - y,
'*': lambda x, y: x * y,
'/': lambda x, y: x / y
}
# 输入数字和运算符
num1 = float(input())
operator = input().strip()
num2 = float(input())
# 根据运算符调用对应的函数进行计算
result = operators[operator](num1, num2)
# 输出结果,保留2位小数
print('%.2f' % result)
```
运行程序后,依次输入两个数字和一个四则运算符,程序将会输出运算结果,小数保留2位。
相关问题
四则运算(用字典实现),比较python语言的if多分支语句。输入格式:在一行中输入一个数字,在一行中输入一个四则运算符(+,-,*,/)在一行中输入一个数字。输出格式:在一行中输出运算结果(小数保留2位)
抱歉,我可以回答这个问题。以下是 Python 代码实现:
operator_dict = {
"+": lambda x, y: x + y,
"-": lambda x, y: x - y,
"*": lambda x, y: x * y,
"/": lambda x, y: x / y
}
num1 = float(input())
operator = input()
num2 = float(input())
if operator in operator_dict:
result = operator_dict[operator](num1, num2)
print("{:.2f}".format(result))
else:
print("Invalid operator")
四则运算(用字典实现) 在一行中输入一个数字 在一行中输入一个四则运算符(+,-,*,/) 在一行中输入一个数字 例如 样例输入: 7 / 3 样例输出:2.33
好的,这是一个使用Python实现的四则运算程序。它首先会从用户那里获取两个数字和一个运算符,然后根据运算符进行相应的运算并输出结果。
```python
def calculate(num1, operator, num2):
# 创建一个字典来存储四则运算的规则
operations = {
'+': lambda x, y: x + y,
'-': lambda x, y: x - y,
'*': lambda x, y: x * y,
'/': lambda x, y: x / y if y != 0 else None # 如果除数为0,则返回None
}
# 使用字典中的规则进行运算
result = operations.get(operator)(num1, num2)
return result
# 获取用户输入
num1 = float(input("请输入一个数字:"))
operator = input("请输入一个四则运算符(+,-,*,/):")
num2 = float(input("请输入另一个数字:"))
# 执行运算并输出结果
print("结果:", calculate(num1, operator, num2))
```
在这个程序中,我们首先定义了一个函数`calculate`,它接受三个参数:两个数字和一个运算符。然后,我们创建了一个字典`operations`,其中包含了四则运算的规则。接下来,我们使用字典中的规则对输入的两个数字进行运算,并返回结果。最后,我们获取用户输入的两个数字和一个运算符,并调用`calculate`函数进行运算并输出结果。
阅读全文
相关推荐
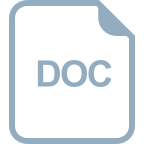
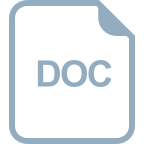
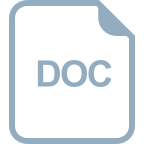


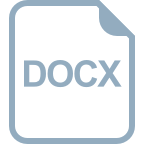
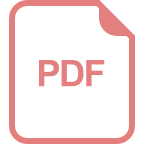
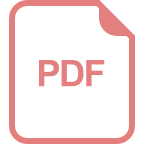
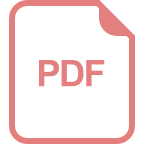
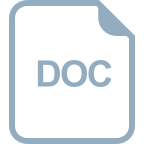
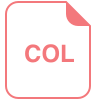
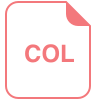
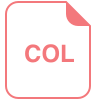
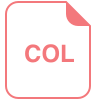
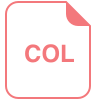

