zint.dll使用
时间: 2024-10-18 07:07:54 浏览: 48
Zint.DLL是一个用于生成高质量PDF条形码的库文件,它通常与.NET平台(如C#、VB.NET等)配合使用。当你需要在应用程序中添加条形码生成功能时,会引用zint.dll来利用其内置的ZINT引擎。这个库支持多种常见的条形码类型,比如Code 128、QR Code等,并且能够定制条形码的样式。
要在.NET项目中使用Zint.dll,你需要执行以下步骤:
1. 将zint.dll文件添加到项目的bin目录或其他合适的托管二进制文件夹中。
2. 引入命名空间:`using Zint;`
3. 创建条形码实例并配置参数,然后调用`Generate`方法生成PDF条形码。
例如,创建一个简单的QR码:
```csharp
string data = "Hello, World!";
var barcode = new Barcode();
barcode.Type = BarcodeType.Qr;
barcode.Data = data;
using (var stream = new MemoryStream())
{
barcode.Write(stream);
// 现在stream里就包含了生成的PDF条形码内容
}
```
相关问题
7z.dll的导出接口没有SevenZipExtract
如果7z.dll的导出接口没有`SevenZipExtract`函数,那么你需要找到正确的函数名称并使用其名称来获取函数指针。7z.dll中有很多不同的函数,根据你的具体情况,你需要找到正确的函数名称。
以下是一个示例代码,使用`GetExportedFunction`函数来获取正确的函数指针:
```cpp
#include <windows.h>
typedef int(__stdcall* SevenZipExtractFunc)(const wchar_t* archiveFile, const wchar_t* destinationPath, const wchar_t* password);
SevenZipExtractFunc GetExportedFunction(HMODULE hModule, const char* functionName)
{
SevenZipExtractFunc functionPtr = NULL;
FARPROC procAddr = GetProcAddress(hModule, functionName);
if (procAddr != NULL)
{
functionPtr = (SevenZipExtractFunc)procAddr;
}
return functionPtr;
}
int main()
{
HINSTANCE hDll = LoadLibrary(L"7z.dll");
if (hDll == NULL)
{
// 无法加载7z.dll
return -1;
}
SevenZipExtractFunc extractFunc = GetExportedFunction(hDll, "FunctionName");
if (extractFunc == NULL)
{
// 无法获取函数指针
FreeLibrary(hDll);
return -1;
}
int result = extractFunc(L"your_tar_file.tar", L"your_extracted_folder", NULL);
FreeLibrary(hDll);
return result;
}
```
在这个示例中,我们定义了一个名为`GetExportedFunction`的函数来获取正确的函数指针。然后,我们使用`GetExportedFunction`函数来获取正确的函数指针,并调用该函数来解压缩文件。
请注意,这只是一个示例代码,你需要根据你的具体情况进行修改。同时,你需要确保7z.dll文件与你的应用程序位于同一目录中,或者将其添加到系统路径中。
希望这可以帮助你解决问题。
c++,如何用7z.dll解压tar.gz压缩包
使用7z.dll解压tar.gz压缩包可以使用C++语言。以下是使用7z.dll解压tar.gz压缩包的代码示例:
```c++
#include "7z.h"
#include "7zAlloc.h"
#include "7zCrc.h"
#include "7zFile.h"
#include "7zVersion.h"
int ExtractTarGZ(const wchar_t* archivePath, const wchar_t* outputFolder)
{
int result = 0;
HANDLE archiveFile = NULL;
HANDLE outputFile = NULL;
wchar_t* archiveFilePath = NULL;
wchar_t* outputFolderPath = NULL;
wchar_t* itemPath = NULL;
SRes res = SZ_OK;
CLookToRead2 lookStream;
CSzArEx db;
ISzAlloc allocImp;
ISzAlloc allocTempImp;
allocImp.Alloc = SzAlloc;
allocImp.Free = SzFree;
allocTempImp.Alloc = SzAllocTemp;
allocTempImp.Free = SzFreeTemp;
CrcGenerateTable();
archiveFilePath = (wchar_t*)malloc(sizeof(wchar_t) * (wcslen(archivePath) + 1));
wcscpy_s(archiveFilePath, wcslen(archivePath) + 1, archivePath);
outputFolderPath = (wchar_t*)malloc(sizeof(wchar_t) * (wcslen(outputFolder) + 1));
wcscpy_s(outputFolderPath, wcslen(outputFolder) + 1, outputFolder);
archiveFile = CreateFileW(archiveFilePath, GENERIC_READ, FILE_SHARE_READ, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (archiveFile == INVALID_HANDLE_VALUE)
{
result = GetLastError();
goto done;
}
lookStream.s.Read = SzFileRead;
lookStream.s.Seek = SzFileSeek;
lookStream.pos = 0;
lookStream.size = 0;
lookStream.object = archiveFile;
lookStream.buf = (Byte*)malloc(kInputBufSize);
SzArEx_Init(&db);
res = SzArEx_Open(&db, &lookStream.s, &allocImp, &allocTempImp);
if (res != SZ_OK)
{
result = res;
goto done;
}
for (UInt32 i = 0; i < db.NumFiles; i++)
{
size_t offset = 0;
size_t outSizeProcessed = 0;
size_t len = SzArEx_GetFileNameUtf16(&db, i, NULL);
itemPath = (wchar_t*)malloc(sizeof(wchar_t) * len);
SzArEx_GetFileNameUtf16(&db, i, itemPath);
if (wcsstr(itemPath, L".tar") != NULL || wcsstr(itemPath, L".gz") != NULL)
{
continue;
}
wchar_t* fullOutputPath = (wchar_t*)malloc(sizeof(wchar_t) * (wcslen(outputFolderPath) + wcslen(itemPath) + 2));
wcscpy_s(fullOutputPath, wcslen(outputFolderPath) + 1, outputFolderPath);
wcscat_s(fullOutputPath, wcslen(outputFolderPath) + wcslen(itemPath) + 2, L"\\");
wcscat_s(fullOutputPath, wcslen(outputFolderPath) + wcslen(itemPath) + 2, itemPath);
if (db.Blocks[i].UnpackSize == 0)
{
outputFile = CreateFileW(fullOutputPath, GENERIC_WRITE, FILE_SHARE_WRITE, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (outputFile == INVALID_HANDLE_VALUE)
{
result = GetLastError();
goto done;
}
CloseHandle(outputFile);
continue;
}
Byte* outBuffer = (Byte*)malloc(db.Blocks[i].UnpackSize);
res = SzArEx_Extract(&db, &lookStream.s, i, &offset, outBuffer, db.Blocks[i].UnpackSize, &outSizeProcessed, &allocTempImp, &allocImp);
if (res != SZ_OK)
{
result = res;
free(outBuffer);
goto done;
}
outputFile = CreateFileW(fullOutputPath, GENERIC_WRITE, FILE_SHARE_WRITE, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (outputFile == INVALID_HANDLE_VALUE)
{
result = GetLastError();
free(outBuffer);
goto done;
}
DWORD bytesWritten = 0;
WriteFile(outputFile, outBuffer, outSizeProcessed, &bytesWritten, NULL);
CloseHandle(outputFile);
free(outBuffer);
free(fullOutputPath);
free(itemPath);
}
done:
if (archiveFile != NULL)
{
CloseHandle(archiveFile);
}
if (lookStream.buf != NULL)
{
free(lookStream.buf);
}
if (archiveFilePath != NULL)
{
free(archiveFilePath);
}
if (outputFolderPath != NULL)
{
free(outputFolderPath);
}
SzArEx_Free(&db, &allocImp);
CrcFreeTable();
return result;
}
```
其中,`archivePath`为tar.gz压缩包的完整路径,`outputFolder`为解压缩后文件的保存目录。这段代码会将压缩包中的所有文件解压到`outputFolder`目录中,忽略`.tar`和`.gz`文件。
阅读全文
相关推荐
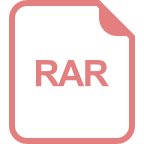
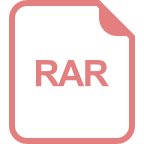
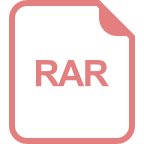
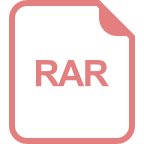
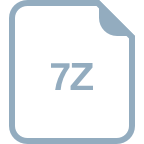
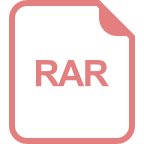
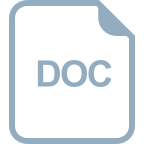
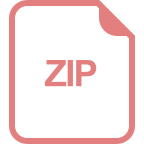
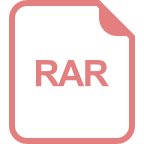
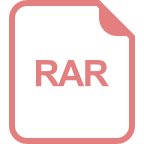
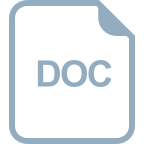
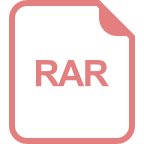



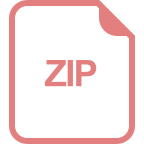
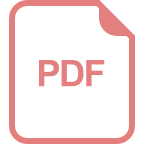