请使用Android Studio实现以下要求,给出范例代码:请添加QQ的登录页面。在登录页面中,如果用户输入的用户名和密码正确,则跳转至如上图所示的好友列表,并记录用户的登录信息,在用户第一次登录成功后再次打开App时,直接登录进入到好友列表页面;如果用户输入的用户名和密码错误,则以Toast方式提示用户输入的用户名或密码错误。 成功登录进入后,侧滑菜单要求仍然可用。 使用SQLite构建内联数据库,其中存储好友基本信息(好友头像、好友名称、最后一次联系的单条消息、最后一次联系的时间)。
时间: 2024-02-27 22:52:34 浏览: 82
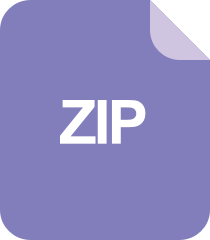
利用Android Studio实现登录界面

好的,以下是实现上述要求的代码示例:
1. 在布局文件中添加登录页面的控件:
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<EditText
android:id="@+id/et_username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入用户名"/>
<EditText
android:id="@+id/et_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:hint="请输入密码"/>
<Button
android:id="@+id/btn_login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="登录"/>
</LinearLayout>
```
2. 在Activity中使用SQLite数据库保存好友信息,并在登录成功后跳转至好友列表页面:
```
public class LoginActivity extends AppCompatActivity {
private EditText etUsername;
private EditText etPassword;
private Button btnLogin;
private SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
etUsername = findViewById(R.id.et_username);
etPassword = findViewById(R.id.et_password);
btnLogin = findViewById(R.id.btn_login);
// 创建或打开数据库
db = openOrCreateDatabase("friends.db", Context.MODE_PRIVATE, null);
// 创建好友信息表
db.execSQL("CREATE TABLE IF NOT EXISTS friends (_id INTEGER PRIMARY KEY AUTOINCREMENT, " +
"name TEXT, avatar BLOB, last_message TEXT, last_contact_time INTEGER)");
// 设置登录按钮的点击事件
btnLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = etUsername.getText().toString();
String password = etPassword.getText().toString();
if (checkUser(username, password)) {
// 保存登录信息
SharedPreferences sp = getSharedPreferences("user", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sp.edit();
editor.putString("username", username);
editor.putString("password", password);
editor.apply();
// 跳转至好友列表页面
Intent intent = new Intent(LoginActivity.this, FriendListActivity.class);
startActivity(intent);
finish();
} else {
Toast.makeText(LoginActivity.this, "用户名或密码错误", Toast.LENGTH_SHORT).show();
}
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
// 关闭数据库
db.close();
}
/**
* 检查用户名和密码是否正确
*/
private boolean checkUser(String username, String password) {
// TODO: 实现检查用户名和密码的逻辑
return true;
}
}
```
3. 在好友列表页面中实现侧滑菜单,并从SQLite数据库中读取好友信息:
```
public class FriendListActivity extends AppCompatActivity {
private DrawerLayout drawerLayout;
private NavigationView navigationView;
private ListView listView;
private SQLiteDatabase db;
private List<Friend> friendList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_friend_list);
drawerLayout = findViewById(R.id.drawer_layout);
navigationView = findViewById(R.id.nav_view);
listView = findViewById(R.id.list_view);
// 创建或打开数据库
db = openOrCreateDatabase("friends.db", Context.MODE_PRIVATE, null);
// 从数据库中读取好友列表
friendList = new ArrayList<>();
Cursor cursor = db.rawQuery("SELECT * FROM friends", null);
while (cursor.moveToNext()) {
Friend friend = new Friend();
friend.setId(cursor.getInt(cursor.getColumnIndex("_id")));
friend.setName(cursor.getString(cursor.getColumnIndex("name")));
friend.setAvatar(cursor.getBlob(cursor.getColumnIndex("avatar")));
friend.setLastMessage(cursor.getString(cursor.getColumnIndex("last_message")));
friend.setLastContactTime(cursor.getLong(cursor.getColumnIndex("last_contact_time")));
friendList.add(friend);
}
cursor.close();
// 设置侧滑菜单的点击事件
navigationView.setNavigationItemSelectedListener(new NavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem item) {
switch (item.getItemId()) {
case R.id.nav_profile:
// TODO: 跳转至个人资料页面
break;
case R.id.nav_settings:
// TODO: 跳转至设置页面
break;
}
drawerLayout.closeDrawer(GravityCompat.START);
return true;
}
});
// 设置ListView的适配器
FriendAdapter adapter = new FriendAdapter(this, friendList);
listView.setAdapter(adapter);
}
@Override
protected void onDestroy() {
super.onDestroy();
// 关闭数据库
db.close();
}
}
```
4. 在AndroidManifest.xml文件中添加权限和Activity声明:
```
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".LoginActivity"/>
<activity android:name=".FriendListActivity"/>
</application>
```
以上是实现该要求的部分示例代码,仅供参考。具体实现还需要根据实际需求进行调整和完善。
阅读全文
相关推荐
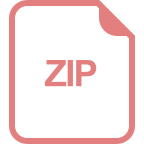
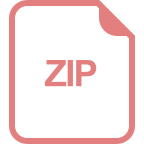

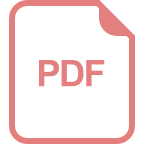
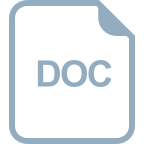
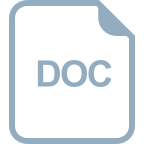









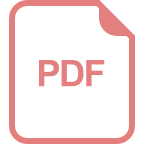
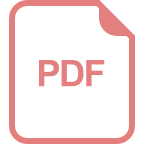
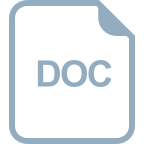