use C program Write a function digit(n, k) that returns the k th digit (from the right) in n (a positive integer). For example digit(829, 1) returns 9, digit(829, 2) returns 2 and digit(829, 3) returns 8. if k is greater than the number of digits in n , have the function return 0.
时间: 2023-12-28 15:04:55 浏览: 42
Sure, here's a C program that defines a function `digit(n, k)` that returns the `k`th digit (from the right) in `n` (a positive integer):
```c
#include <stdio.h>
int digit(int n, int k) {
int count = 0;
while (n > 0) {
count++;
if (count == k) {
return n % 10;
}
n /= 10;
}
return 0;
}
int main() {
int n = 829;
int k = 1;
printf("The %dth digit (from the right) in %d is %d\n", k, n, digit(n, k));
k = 2;
printf("The %dth digit (from the right) in %d is %d\n", k, n, digit(n, k));
k = 3;
printf("The %dth digit (from the right) in %d is %d\n", k, n, digit(n, k));
k = 4;
printf("The %dth digit (from the right) in %d is %d\n", k, n, digit(n, k));
return 0;
}
```
This program defines a function `digit(n, k)` that takes two arguments: `n` (the positive integer) and `k` (the index of the digit to return, from the right). The function works by counting the number of digits in `n` as it repeatedly divides `n` by 10. If `count` equals `k`, the function returns the last digit of `n`. If `count` exceeds the number of digits in `n`, the function returns 0.
I hope that helps! Let me know if you have any further questions.
相关推荐
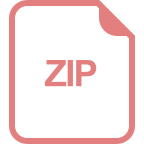
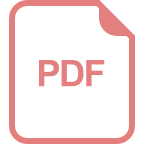














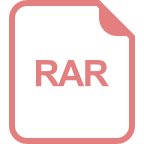