用java写一个动态流星雨
时间: 2024-10-23 10:06:34 浏览: 88
在Java中创建一个动态流星雨效果通常涉及到图形界面、动画和随机数生成。以下是一个简单的例子,我们将使用Swing库来绘制流星,由于文字描述限制,这里提供一个简化的伪代码框架:
```java
import javax.swing.*;
import java.awt.*;
import java.util.Random;
public class MeteorRain extends JFrame {
private static final int NUM_METEORS = 50;
private JPanel canvas;
private Random random;
public MeteorRain() {
// 初始化窗口
setTitle("流星雨");
setSize(800, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 创建画布并添加到窗口
canvas = new JPanel();
canvas.setBackground(Color.BLACK); // 黑色背景突出流星
add(canvas);
// 设置随机数生成器
random = new Random();
// 开始绘制动画
startAnimation();
}
private void startAnimation() {
Timer timer = new Timer(100, e -> drawMeteor());
timer.start();
}
private void drawMeteor() {
for (int i = 0; i < NUM_METEORS; i++) {
int x = random.nextInt(getWidth());
int y = getHeight() - random.nextInt(500); // 每次下降一点直到消失
canvas.add(new Meteor(x, y)); // 添加流星到画布
// 清除已过期的流星,这里可以用Stack或List替换数组来实现
if (canvas.getComponentCount() > NUM_METEORS * 2) {
Component c = canvas.getComponent(0);
canvas.remove(c);
}
}
}
// Meteor类,包含简单绘图方法
class Meteor extends JPanel {
int x, y;
public Meteor(int x, int y) {
this.x = x;
this.y = y;
setPreferredSize(new Dimension(5, 5));
setLocation(x, y);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.WHITE); // 流星颜色
g.fillRect(x, y, getWidth(), getHeight()); // 绘制流星
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
new MeteorRain().setVisible(true);
});
}
}
```
这个代码中,`MeteorRain` 类负责整个窗口的管理,以及启动流星动画。每个 `Meteor` 对象代表一颗流星,它们在窗口上随机位置落下,然后消失。
注意,这只是一个基础的示例,实际应用中可能会使用更复杂的图形库如JavaFX或LWJGL来实现更流畅的动画效果。此外,如果你希望在屏幕上看到真正的流星效果,可能需要引入一些视觉效果的优化和处理,例如粒子系统。
阅读全文
相关推荐
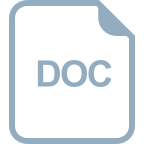
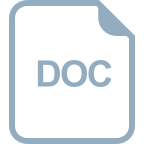
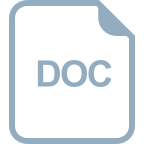
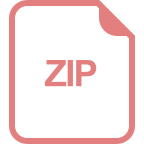
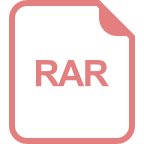
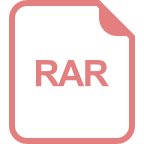


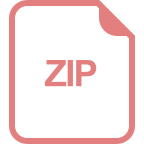
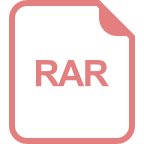




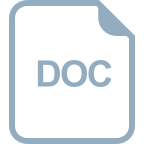
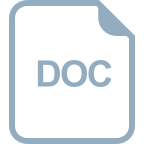