485 232串口参数配置c语言
时间: 2023-08-24 17:28:46 浏览: 85
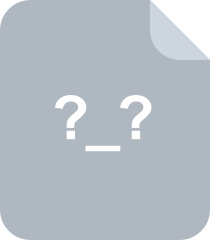
c语言操作串口支持485/232
串口参数配置主要包括波特率、数据位、停止位和校验位等。下面是一个示例代码,可以配置485和232两种串口的参数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define PORT_485 "/dev/ttyUSB0"
#define PORT_232 "/dev/ttyUSB1"
int set_serial(int fd, int baud_rate, int data_bits, char parity, int stop_bits)
{
struct termios options;
// 获取当前配置
tcgetattr(fd, &options);
// 设置波特率
switch (baud_rate)
{
case 9600:
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
break;
case 19200:
cfsetispeed(&options, B19200);
cfsetospeed(&options, B19200);
break;
case 38400:
cfsetispeed(&options, B38400);
cfsetospeed(&options, B38400);
break;
case 57600:
cfsetispeed(&options, B57600);
cfsetospeed(&options, B57600);
break;
case 115200:
cfsetispeed(&options, B115200);
cfsetospeed(&options, B115200);
break;
default:
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
break;
}
// 设置数据位
options.c_cflag &= ~CSIZE;
switch (data_bits)
{
case 5:
options.c_cflag |= CS5;
break;
case 6:
options.c_cflag |= CS6;
break;
case 7:
options.c_cflag |= CS7;
break;
case 8:
options.c_cflag |= CS8;
break;
default:
options.c_cflag |= CS8;
break;
}
// 设置校验位
switch (parity)
{
case 'n':
case 'N':
options.c_cflag &= ~PARENB; // Clear parity enable
options.c_iflag &= ~INPCK; // Enable parity checking
break;
case 'o':
case 'O':
options.c_cflag |= (PARODD | PARENB); // 设置为奇校验
options.c_iflag |= INPCK; // Disable parity checking
break;
case 'e':
case 'E':
options.c_cflag |= PARENB; // Enable parity
options.c_cflag &= ~PARODD; // 转换为偶校验
options.c_iflag |= INPCK; // Disable parity checking
break;
default:
options.c_cflag &= ~PARENB; // Clear parity enable
options.c_iflag &= ~INPCK; // Enable parity checking
break;
}
// 设置停止位
switch (stop_bits)
{
case 1:
options.c_cflag &= ~CSTOPB;
break;
case 2:
options.c_cflag |= CSTOPB;
break;
default:
options.c_cflag &= ~CSTOPB;
break;
}
// 设置控制模式
options.c_cflag |= CLOCAL | CREAD;
// 设置输入模式
options.c_iflag &= ~(IXON | IXOFF | IXANY); // 禁止软件流控
// 设置输出模式
options.c_oflag &= ~OPOST;
// 设置等待时间和最小字符数
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
// 清空串口输入输出缓存区
tcflush(fd, TCIFLUSH);
tcflush(fd, TCOFLUSH);
// 应用配置
if (tcsetattr(fd, TCSANOW, &options) != 0)
{
return -1;
}
return 0;
}
int main()
{
int fd485, fd232;
char buf[1024];
int n;
// 打开485串口
if ((fd485 = open(PORT_485, O_RDWR | O_NOCTTY | O_NONBLOCK)) < 0)
{
perror("open 485 error");
exit(1);
}
// 配置485串口
if (set_serial(fd485, 115200, 8, 'N', 1) < 0)
{
perror("set 485 error");
close(fd485);
exit(1);
}
// 打开232串口
if ((fd232 = open(PORT_232, O_RDWR | O_NOCTTY | O_NONBLOCK)) < 0)
{
perror("open 232 error");
close(fd485);
exit(1);
}
// 配置232串口
if (set_serial(fd232, 115200, 8, 'N', 1) < 0)
{
perror("set 232 error");
close(fd485);
close(fd232);
exit(1);
}
// 读取485串口数据
n = read(fd485, buf, sizeof(buf));
if (n > 0)
{
printf("485: %s\n", buf);
}
// 读取232串口数据
n = read(fd232, buf, sizeof(buf));
if (n > 0)
{
printf("232: %s\n", buf);
}
// 关闭串口
close(fd485);
close(fd232);
return 0;
}
```
需要注意的是,串口参数的设置应该与设备的实际要求相匹配,否则会导致通讯失败。
阅读全文
相关推荐
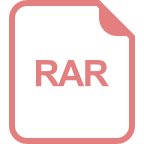
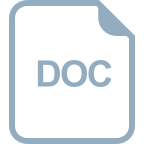
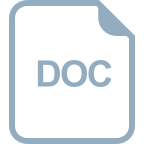
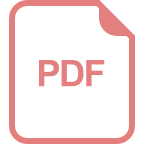
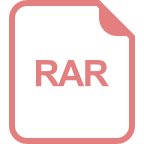
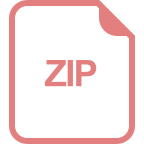
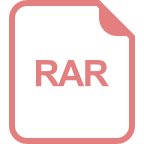
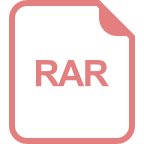
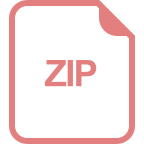
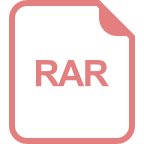
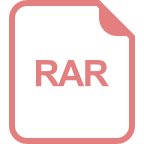
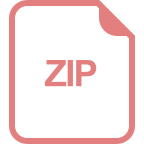
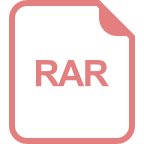
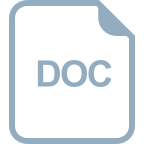
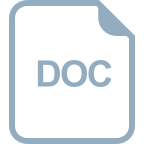
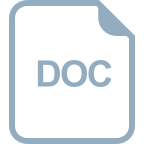
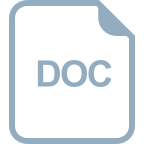