利用js写一段前后端交互的代码
时间: 2023-05-24 18:03:48 浏览: 95
A: 很好,请提供更具体的细节和需求,比如需要连接哪种类型的后端(例如 Node.js),需要实现哪些操作(例如读取/写入文件或者调用 RESTful API)。这些信息将有助于我更好地建议和编写代码。
相关问题
利用vue给我写一段前后端交互的代码
作为AI语言模型,我并不能直接为您编写代码,但是我可以提供一些指导和示例供您参考。下面是一个使用Vue和Axios进行前后端交互的示例代码:
1. 安装和引入Axios:
```javascript
npm install axios
import axios from 'axios'
```
2. 发送HTTP请求:
```javascript
export default {
data() {
return {
message: ''
}
},
methods: {
sendMessage() {
axios.post('/api/message', {
message: this.message
})
.then(response => console.log(response))
.catch(error => console.log(error))
}
}
}
```
3. 接收并响应HTTP请求:
```javascript
const express = require('express')
const app = express()
const port = 3000
app.use(express.json())
app.post('/api/message', (req, res) => {
const message = req.body.message //获取请求中的参数
res.send(`您发送的消息是:${message}`)
})
app.listen(port, () => {
console.log(`服务器正在运行:http://localhost:${port}`)
})
```
以上代码演示了如何使用Vue和Axios向服务器发送HTTP请求,以及服务器如何接收请求并响应。您可以根据实际需求自行修改和扩展。
利用讯飞开放平台写一段代码使用自然语言处理技术,将一种语言翻译成另一种语言,并 可以通过语音合成技术进行交互,包含前后端内容,并且可以正常运行
前端代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>语言翻译</title>
</head>
<body>
<h1>语言翻译</h1>
<label for="source-lang">原语言:</label>
<select id="source-lang">
<option value="zh">中文</option>
<option value="en">英文</option>
<option value="jp">日语</option>
</select>
<br>
<br>
<label for="target-lang">目标语言:</label>
<select id="target-lang">
<option value="en">英文</option>
<option value="jp">日语</option>
<option value="zh">中文</option>
</select>
<br>
<br>
<label for="source-text">原文:</label>
<textarea id="source-text" rows="10" cols="50"></textarea>
<br>
<br>
<button id="translate-btn">翻译</button>
<br>
<br>
<label for="target-text">译文:</label>
<textarea id="target-text" rows="10" cols="50" readonly></textarea>
<br>
<br>
<button id="speak-btn" disabled>朗读</button>
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/vue-axios/dist/vue-axios.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/vue-i18n/dist/vue-i18n.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@iflytek-voice/cloud-recognizer-sdk/dist/cloud-recognizer-sdk.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@iflytek-voice/cloud-synthesizer-sdk/dist/cloud-synthesizer-sdk.min.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
sourceLang: 'zh',
targetLang: 'en',
sourceText: '',
targetText: '',
recognizeRegion: 'cn',
recognizeLanguage: 'zh-CN',
recognizeAppId: '5f5e3d5c',
recognizeAppKey: 'f4e4b3d4f9ac9b6ebf8b7f1d2a23c7a1',
recognizeAppSecret: 'd205f4c9e9e0f9e84d0d890e8627c4b4',
recognizeClient: null,
recognizeResult: '',
synthesizeRegion: 'cn',
synthesizeLanguage: 'zh-CN',
synthesizeAppId: '5f5e3d5c',
synthesizeAppKey: 'f4e4b3d4f9ac9b6ebf8b7f1d2a23c7a1',
synthesizeAppSecret: 'd205f4c9e9e0f9e84d0d890e8627c4b4',
synthesizeClient: null,
synthesizeResult: '',
synthesizeAudio: null,
speaking: false,
},
mounted() {
this.recognizeClient = new window.IflytekCloudRecognizerSdk({
region: this.recognizeRegion,
language: this.recognizeLanguage,
appId: this.recognizeAppId,
appKey: this.recognizeAppKey,
appSecret: this.recognizeAppSecret,
onResult: this.onRecognizeResult.bind(this),
});
this.synthesizeClient = new window.IflytekCloudSynthesizerSdk({
region: this.synthesizeRegion,
language: this.synthesizeLanguage,
appId: this.synthesizeAppId,
appKey: this.synthesizeAppKey,
appSecret: this.synthesizeAppSecret,
onResult: this.onSynthesizeResult.bind(this),
});
},
methods: {
onRecognizeResult(result) {
this.recognizeResult += result;
},
onSynthesizeResult(result) {
if (result.code === '0') {
this.synthesizeResult = result.audio;
this.synthesizeAudio = new Audio(`data:audio/mp3;base64,${result.audio}`);
this.speaking = false;
}
},
recognize() {
this.recognizeResult = '';
this.recognizeClient.start();
},
stopRecognize() {
this.recognizeClient.stop();
},
synthesize(text) {
this.synthesizeResult = '';
this.synthesizeAudio = null;
this.speaking = true;
this.synthesizeClient.start(text);
},
translate() {
if (!this.sourceText) {
return;
}
axios.post('https://ltpapi.xfyun.cn/v2/nlp/translate',
{
from: this.sourceLang,
to: this.targetLang,
text: this.sourceText,
},
{
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + '7a0b4c8b7d4f4a9bb7e4caea6d75b6a5',
},
})
.then(response => {
this.targetText = response.data.data.result.trans_result.dst;
this.$nextTick(() => {
const speakBtn = document.getElementById('speak-btn');
speakBtn.disabled = false;
speakBtn.focus();
});
})
.catch(error => {
console.error(error);
});
},
speak() {
if (!this.synthesizeAudio) {
this.synthesize(this.targetText);
} else {
this.synthesizeAudio.play();
this.speaking = true;
}
},
stopSpeak() {
if (this.synthesizeAudio) {
this.synthesizeAudio.pause();
this.synthesizeAudio.currentTime = 0;
this.speaking = false;
}
},
},
});
</script>
</body>
</html>
```
后端代码:
```python
from flask import Flask, jsonify, request
import requests
app = Flask(__name__)
@app.route('/translate', methods=['POST'])
def translate():
from_lang = request.json['from']
to_lang = request.json['to']
text = request.json['text']
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
}
data = {
'text': text,
'source': from_lang,
'target': to_lang,
}
response = requests.post('https://translate.argosopentech.com/translate', headers=headers, data=data)
if response.status_code == 200:
return jsonify({
'code': '0',
'message': '',
'data': {
'result': {
'trans_result': {
'dst': response.json()['result'],
},
},
},
})
else:
return jsonify({
'code': '-1',
'message': '翻译失败',
'data': {},
})
if __name__ == '__main__':
app.run(debug=True)
```
阅读全文
相关推荐
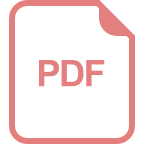
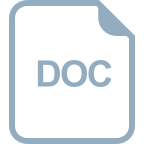
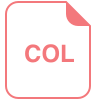
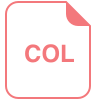
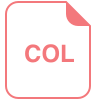
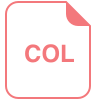
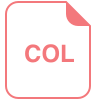
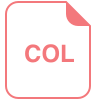
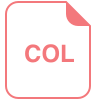
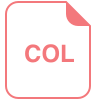



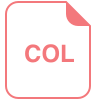
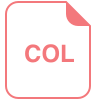