写一个例子__android_log_print
时间: 2023-05-12 08:03:25 浏览: 283
__android_log_print是一个用于在Android平台上打印日志的函数,其原型如下:
void __android_log_print(int prio, const char *tag, const char *fmt, ...);
其中,prio表示日志的优先级,tag表示日志的标签,fmt表示日志的格式,...表示可变参数列表。
下面是一个使用__android_log_print函数打印日志的例子:
#include <android/log.h>
#define TAG "MyApp"
void printLog(const char* message) {
__android_log_print(ANDROID_LOG_DEBUG, TAG, "%s", message);
}
在这个例子中,我们定义了一个printLog函数,它接受一个字符串参数message,并使用__android_log_print函数打印日志。优先级为ANDROID_LOG_DEBUG,标签为"MyApp",格式为"%s",表示输出message的值。
注意:在使用__android_log_print函数之前,需要包含<android/log.h>头文件。
相关问题
android c++ log输出
在Android中,如果你想要在C++代码中进行日志输出,通常会使用Android的日志系统`android.util.Log`。这个系统提供了一套方便的方法来记录应用程序运行过程中的信息、警告和错误。
以下是一个基本的例子:
```cpp
#include <android/log.h>
#define LOG_TAG "MyApp" // 标识你的log条目所属的应用标签
#define LOGI(...) __android_log_print(ANDROID_LOG_INFO, LOG_TAG, __VA_ARGS__) // 输出info级别日志
#define LOGW(...) __android_log_print(ANDROID_LOG_WARN, LOG_TAG, __VA_ARGS__) // 输出warn级别日志
#define LOGE(...) __android_log_print(ANDROID_LOG_ERROR, LOG_TAG, __VA_ARGS__) // 输出error级别日志
// 使用示例
LOGI("This is an info message");
LOGW("A warning occurred");
LOGE("An error has happened");
```
在这个例子中,`__android_log_print`是一个宏,它会将消息打印到Logcat,这是开发者常用的调试工具。你需要替换`LOG_TAG`为你应用的实际tag,便于在Logcat中过滤和查找相应的log。
Android 使用c++MediaCodec 解码例子
以下是使用C++ MediaCodec 进行视频解码的示例代码:
```c++
#include <jni.h>
#include <android/log.h>
#include <android/native_window_jni.h>
#include <media/NdkMediaCodec.h>
#include <media/NdkMediaExtractor.h>
#define LOG_TAG "MediaCodec"
#define LOGI(...) __android_log_print(ANDROID_LOG_INFO, LOG_TAG, __VA_ARGS__)
#define LOGE(...) __android_log_print(ANDROID_LOG_ERROR, LOG_TAG, __VA_ARGS__)
extern "C" JNIEXPORT void JNICALL
Java_com_example_mediadecoder_MainActivity_decode(JNIEnv *env, jobject thiz, jstring filePath, jobject surface) {
const char *path = env->GetStringUTFChars(filePath, nullptr);
AMediaExtractor *extractor = AMediaExtractor_new();
AMediaCodec *codec = nullptr;
int32_t trackIndex = -1;
bool sawInputEOS = false;
bool sawOutputEOS = false;
// 设置文件路径
media_status_t result = AMediaExtractor_setDataSource(extractor, path);
if (result != AMEDIA_OK) {
LOGE("AMediaExtractor_setDataSource error: %d", result);
goto end;
}
// 查找视频轨道
int32_t numTracks = AMediaExtractor_getTrackCount(extractor);
for (int32_t i = 0; i < numTracks; ++i) {
AMediaFormat *format = AMediaExtractor_getTrackFormat(extractor, i);
const char *mime = nullptr;
AMediaFormat_getString(format, AMEDIAFORMAT_KEY_MIME, &mime);
if (mime && !strncmp(mime, "video/", 6)) {
trackIndex = i;
break;
}
AMediaFormat_delete(format);
}
if (trackIndex == -1) {
LOGE("No video track found in %s", path);
goto end;
}
// 获取视频格式
AMediaFormat *format = AMediaExtractor_getTrackFormat(extractor, trackIndex);
int32_t width = 0, height = 0;
AMediaFormat_getInt32(format, AMEDIAFORMAT_KEY_WIDTH, &width);
AMediaFormat_getInt32(format, AMEDIAFORMAT_KEY_HEIGHT, &height);
LOGI("Video size: %dx%d", width, height);
// 创建 MediaCodec 解码器
codec = AMediaCodec_createDecoderByType("video/avc");
result = AMediaCodec_configure(codec, format, ANativeWindow_fromSurface(env, surface), nullptr, 0);
if (result != AMEDIA_OK) {
LOGE("AMediaCodec_configure error: %d", result);
goto end;
}
// 启动解码器
result = AMediaCodec_start(codec);
if (result != AMEDIA_OK) {
LOGE("AMediaCodec_start error: %d", result);
goto end;
}
// 解码视频帧
AMediaCodecBufferInfo bufferInfo;
int64_t presentationTimeUs;
size_t bufIdx;
while (!sawOutputEOS) {
if (!sawInputEOS) {
bufIdx = AMediaExtractor_getSampleTrackIndex(extractor);
if (bufIdx == trackIndex) {
result = AMediaExtractor_readSampleData(extractor, AMediaCodec_getInputBuffer(codec, bufIdx), 0);
if (result == AMEDIA_OK) {
presentationTimeUs = AMediaExtractor_getSampleTime(extractor);
AMediaCodec_queueInputBuffer(codec, bufIdx, 0, AMediaExtractor_getSampleSize(extractor), presentationTimeUs,
AMediaExtractor_getSampleFlags(extractor));
AMediaExtractor_advance(extractor);
} else if (result == AMEDIA_ERROR_END_OF_STREAM) {
sawInputEOS = true;
AMediaCodec_queueInputBuffer(codec, bufIdx, 0, 0, 0, AMEDIACODEC_BUFFER_FLAG_END_OF_STREAM);
} else {
LOGE("AMediaExtractor_readSampleData error: %d", result);
goto end;
}
}
}
result = AMediaCodec_dequeueOutputBuffer(codec, &bufferInfo, 5000);
if (result == AMEDIA_OK) {
if (bufferInfo.flags & AMEDIACODEC_BUFFER_FLAG_END_OF_STREAM) {
sawOutputEOS = true;
}
AMediaCodec_releaseOutputBuffer(codec, result, true);
} else if (result == AMEDIACODEC_INFO_OUTPUT_FORMAT_CHANGED) {
AMediaFormat *newFormat = nullptr;
newFormat = AMediaCodec_getOutputFormat(codec);
AMediaFormat_getInt32(newFormat, AMEDIAFORMAT_KEY_WIDTH, &width);
AMediaFormat_getInt32(newFormat, AMEDIAFORMAT_KEY_HEIGHT, &height);
LOGI("Video output format changed to %dx%d", width, height);
AMediaFormat_delete(newFormat);
} else if (result == AMEDIACODEC_INFO_OUTPUT_BUFFERS_CHANGED) {
LOGI("AMediaCodec_dequeueOutputBuffer returned AMEDIACODEC_INFO_OUTPUT_BUFFERS_CHANGED");
} else {
LOGE("AMediaCodec_dequeueOutputBuffer error: %d", result);
goto end;
}
}
end:
if (codec) {
AMediaCodec_stop(codec);
AMediaCodec_delete(codec);
}
if (extractor) {
AMediaExtractor_delete(extractor);
}
env->ReleaseStringUTFChars(filePath, path);
}
```
在此示例代码中,我们使用 `AMediaExtractor` 获取视频文件中的视频轨道,并使用 `AMediaCodec` 进行解码。注意,此代码仅支持解码 H.264 编码视频。
阅读全文
相关推荐
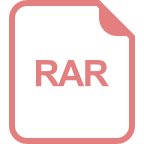
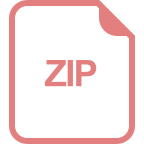
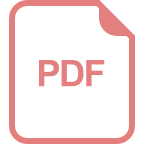
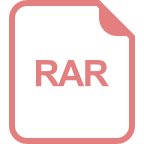
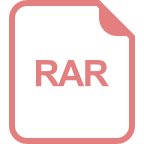
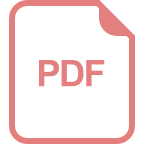
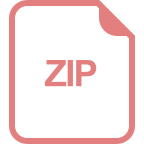
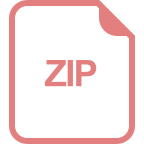
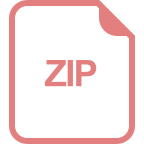
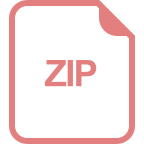
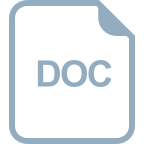
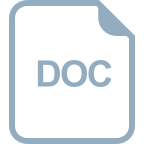
