RecyclerView边缘渐隐
时间: 2023-11-16 19:54:15 浏览: 35
可以通过设置RecyclerView的ItemDecoration来实现边缘渐隐效果。具体实现步骤如下:
1. 自定义一个继承自RecyclerView.ItemDecoration的类,重写其中的getItemOffsets()和onDraw()方法。
2. 在getItemOffsets()方法中,根据item的位置设置不同方向的偏移量,以便在onDraw()方法中绘制出边缘渐隐的效果。
3. 在onDraw()方法中,根据item的位置和偏移量绘制出边缘渐隐的效果。
以下是一个简单的示例代码:
```
public class EdgeFadeItemDecoration extends RecyclerView.ItemDecoration {
private static final int DEFAULT_FADE_SIZE = 32; // 默认渐隐大小
private int mFadeSize; // 渐隐大小
public EdgeFadeItemDecoration() {
this(DEFAULT_FADE_SIZE);
}
public EdgeFadeItemDecoration(int fadeSize) {
mFadeSize = fadeSize;
}
@Override
public void getItemOffsets(Rect outRect, View view, RecyclerView parent, RecyclerView.State state) {
int position = parent.getChildAdapterPosition(view);
int itemCount = parent.getAdapter().getItemCount();
if (position == 0) { // 第一个item
outRect.set(0, mFadeSize, 0, 0);
} else if (position == itemCount - 1) { // 最后一个item
outRect.set(0, 0, 0, mFadeSize);
} else { // 中间的item
outRect.set(0, 0, 0, 0);
}
}
@Override
public void onDraw(Canvas c, RecyclerView parent, RecyclerView.State state) {
int childCount = parent.getChildCount();
int itemCount = parent.getAdapter().getItemCount();
int left = parent.getPaddingLeft();
int right = parent.getWidth() - parent.getPaddingRight();
for (int i = 0; i < childCount; i++) {
View child = parent.getChildAt(i);
int position = parent.getChildAdapterPosition(child);
if (position == 0) { // 第一个item
int top = child.getTop() - mFadeSize;
int bottom = child.getTop();
drawFade(c, left, top, right, bottom);
} else if (position == itemCount - 1) { // 最后一个item
int top = child.getBottom();
int bottom = child.getBottom() + mFadeSize;
drawFade(c, left, top, right, bottom);
}
}
}
private void drawFade(Canvas c, int left, int top, int right, int bottom) {
GradientDrawable gradientDrawable = new GradientDrawable(GradientDrawable.Orientation.TOP_BOTTOM,
new int[]{Color.TRANSPARENT, Color.BLACK});
gradientDrawable.setBounds(left, top, right, bottom);
gradientDrawable.draw(c);
}
}
```
相关推荐
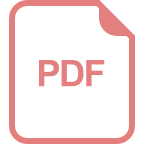
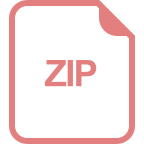



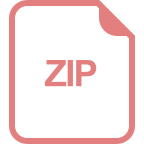
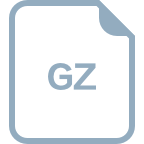
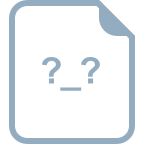
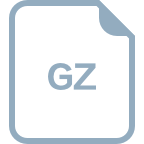
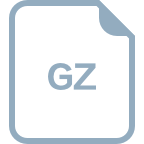