给定一个字符串数组,打印数组中的所有词。请用C代码实现
时间: 2023-03-29 22:01:06 浏览: 129
以下是C代码实现:
#include <stdio.h>
int main() {
char words[][10] = {"hello", "world", "this", "is", "a", "test"}; // 字符串数组
int num_words = sizeof(words) / sizeof(words[]); // 数组中字符串的数量
for (int i = ; i < num_words; i++) {
printf("%s ", words[i]); // 打印每个字符串
}
return ;
}
相关问题
用Java给定[“a”,”b”,"a”,”b”,”c”,”a”,”b’,”c”,”b”字符串数组,然后使用Map的key来保存数组中字符串元素,value保存该字符串元素的出现次数,最后统计出各字符串
在Java中,你可以使用`HashMap`或者`LinkedHashMap`来实现这个需求,因为它们可以存储键值对,并能快速查找和计算每个键的出现次数。下面是一个示例代码:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] array = {"a", "b", "a", "b", "c", "a", "b", "c", "b"};
Map<String, Integer> frequencyMap = new HashMap<>();
for (String s : array) {
if (frequencyMap.containsKey(s)) {
frequencyMap.put(s, frequencyMap.get(s) + 1);
} else {
frequencyMap.put(s, 1);
}
}
// 打印结果
for (Map.Entry<String, Integer> entry : frequencyMap.entrySet()) {
System.out.println("字符串 '" + entry.getKey() + "' 出现了 " + entry.getValue() + " 次");
}
}
}
```
在这个代码中,我们遍历字符串数组,对于每个字符串,如果它已经在`frequencyMap`中,我们就增加对应的计数;如果不在,就添加新的键值对,初始值为1。最后,我们打印出每个字符串及其出现的次数。
用C语言实现:给定一个字符串 其中有些字符在字符串中出现了2次 找到这些字符第一次出现的位置,字符在字符串中的位置从1开始
好的,我能帮你实现这个功能。以下是 C 语言的代码实现:
```
#include <stdio.h>
#include <string.h>
void find_duplicate_chars(char* str) {
int len = strlen(str);
int char_count[256] = {0};
for (int i = 0; i < len; i++) {
char_count[str[i]]++;
}
for (int i = 0; i < len; i++) {
if (char_count[str[i]] == 2) {
printf("Character %c first appears at position %d\n", str[i], i + 1);
char_count[str[i]] = 0;
}
}
}
int main() {
char str[] = "abbcdeffggh";
find_duplicate_chars(str);
return 0;
}
```
代码解释如下:
- `find_duplicate_chars` 函数接收一个字符串作为输入,并将重复出现的字符的第一次出现位置打印出来。
- 在 `find_duplicate_chars` 函数中,首先计算每个字符出现的次数,存储在 `char_count` 数组中。
- 然后遍历字符串中的每个字符,如果这个字符在字符串中出现了两次,就打印出它第一次出现的位置,并将它在 `char_count` 数组中的计数清零。
- 最后在 `main` 函数中调用 `find_duplicate_chars` 函数,传入一个测试字符串,即可测试代码的正确性。
希望这个代码能够帮到你!
阅读全文
相关推荐
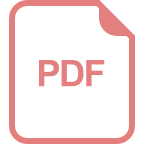
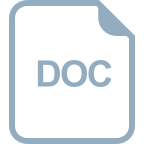
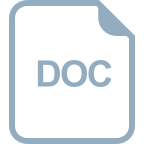
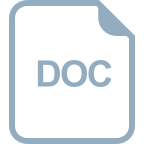
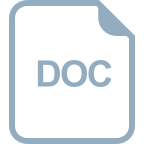
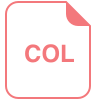





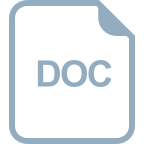


