用python定义一个类MyMath,提供基本的加减乘除功能,并进行测试。
时间: 2023-05-22 18:01:49 浏览: 131
好的,以下是用 Python 定义一个类 MyMath,提供基本的加减乘除功能,并进行测试的代码:
```python
class MyMath:
def add(self, x, y):
return x + y
def subtract(self, x, y):
return x - y
def multiply(self, x, y):
return x * y
def divide(self, x, y):
if y == 0:
raise ValueError("Cannot divide by zero!")
return x / y
# 测试
math = MyMath()
print(math.add(2, 3)) # 输出 5
print(math.subtract(5, 2)) # 输出 3
print(math.multiply(4, 6)) # 输出 24
print(math.divide(10, 2)) # 输出 5.0
```
希望这个代码能够满足您的需求。
相关问题
python定义一个类MyMath,提供基本的加减乘除功能,并进行测试。
好的,以下是定义一个类MyMath并提供基本的加减乘除功能的Python代码:
```
class MyMath:
def add(self, x, y):
return x + y
def subtract(self, x, y):
return x - y
def multiply(self, x, y):
return x * y
def divide(self, x, y):
if y == 0:
raise ValueError("Cannot divide by zero!")
return x / y
# 测试
math = MyMath()
print(math.add(2, 3)) # 输出 5
print(math.subtract(5, 2)) # 输出 3
print(math.multiply(4, 6)) # 输出 24
print(math.divide(10, 2)) # 输出 5.0
```
希望这个代码能够满足您的需求。
3、定义一个类MyMath,提供基本的加减乘除功能,然后进行测试。
好的,这是一个简单的MyMath类的示例代码:
```python
class MyMath:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
def multiply(self, a, b):
return a * b
def divide(self, a, b):
if b == 0:
return "Error: division by zero"
else:
return a / b
```
其中,add、subtract、multiply、divide分别对应加、减、乘、除四种基本运算。
接下来,我们可以进行测试,例如:
```python
# 创建MyMath类的实例
calculator = MyMath()
# 进行加法计算
print(calculator.add(1, 2)) # 输出3
# 进行减法计算
print(calculator.subtract(5, 3)) # 输出2
# 进行乘法计算
print(calculator.multiply(4, 6)) # 输出24
# 进行除法计算
print(calculator.divide(10, 2)) # 输出5.0
print(calculator.divide(10, 0)) # 输出"Error: division by zero"
```
以上就是一个简单的MyMath类的定义和测试。
阅读全文
相关推荐




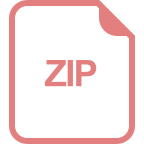







