掉字符串首尾空格c++
时间: 2023-12-02 16:05:44 浏览: 88
可以使用C++中的字符串库函数`std::string::find_first_not_of`和`std::string::find_last_not_of`来完成去掉首尾空格的操作。具体实现如下:
```c++
#include <iostream>
#include <string>
using namespace std;
string trim(string s) {
size_t start = s.find_first_not_of(" ");
size_t end = s.find_last_not_of(" ");
return s.substr(start, end - start + 1);
}
int main() {
string s = " hello world ";
string result = trim(s);
cout << result << endl; // 输出 "hello world"
return 0;
}
```
在上面的代码中,`trim`函数接受一个字符串参数`s`,并返回去掉首尾空格后的字符串。`find_first_not_of`函数用来查找第一个不是空格的字符的位置,`find_last_not_of`函数用来查找最后一个不是空格的字符的位置,然后我们使用`substr`函数从`start`位置开始,长度为`end - start + 1`的子串作为结果返回。
相关问题
掉字符串首尾空格c++ 用指针
可以使用指针来去掉字符串首尾的空格,具体实现如下:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void trim(char* str) {
if (str == nullptr) {
return;
}
// 去掉字符串前面的空格
char* start = str;
while (*start == ' ') {
start++;
}
// 去掉字符串后面的空格
char* end = start + strlen(start) - 1;
while (end > start && *end == ' ') {
end--;
}
*(end + 1) = '\0';
// 拷贝去掉空格后的字符串到原字符串
if (start != str) {
strcpy(str, start);
}
}
int main() {
char str[] = " hello world ";
trim(str);
cout << str << endl; // 输出 hello world
return 0;
}
```
在该代码中,首先定义了一个 `trim` 函数,该函数通过指针遍历字符串,去掉字符串前面和后面的空格,并将去掉空格后的字符串拷贝回原字符串中。最后,在 `main` 函数中测试了一下该函数的功能。
去掉字符串首尾空格C++指针
可以使用C++的字符串库函数`trim`去掉字符串首尾空格,示例代码如下:
```c++
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
int main() {
string str = " hello world ";
str.erase(str.begin(), find_if(str.begin(), str.end(), [](int ch) {
return !isspace(ch);
}));
str.erase(find_if(str.rbegin(), str.rend(), [](int ch) {
return !isspace(ch);
}).base(), str.end());
cout << "去掉首尾空格后的字符串为:" << str << endl;
return 0;
}
```
输出结果为:
```
去掉首尾空格后的字符串为:hello world
```
阅读全文
相关推荐















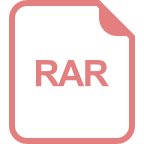