springboot+vue 实现excel数据导入功能
时间: 2023-09-09 18:09:57 浏览: 127
实现 excel 数据导入的功能,需要完成以下步骤:
1. 前端页面实现文件上传功能,可以使用 vue-upload-component 组件实现。
2. 后端使用 Spring Boot 框架,导入 poi 依赖,使用 poi 解析 Excel 文件,获取数据并保存到数据库中。
下面是具体的实现步骤:
1. 前端页面
在 vue 组件中,使用 vue-upload-component 组件实现文件上传功能。具体实现代码如下:
```
<template>
<div>
<input type="file" ref="file" @change="handleFileChange">
<button @click.prevent="submit">上传</button>
</div>
</template>
<script>
import UploadComponent from 'vue-upload-component'
export default {
components: {
UploadComponent
},
data () {
return {
file: null
}
},
methods: {
handleFileChange () {
this.file = this.$refs.file.files[0]
},
submit () {
const formData = new FormData()
formData.append('file', this.file)
this.$http.post('/upload', formData)
.then(res => {
console.log(res)
})
.catch(err => {
console.log(err)
})
}
}
}
</script>
```
2. 后端实现
2.1 添加依赖
在 Spring Boot 项目的 pom.xml 文件中添加 poi 依赖:
```
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
```
2.2 实现文件上传接口
在 Spring Boot 项目中,实现文件上传的接口:
```
@PostMapping("/upload")
public void upload(MultipartFile file) throws Exception {
Workbook workbook = WorkbookFactory.create(file.getInputStream());
Sheet sheet = workbook.getSheetAt(0);
List<Data> dataList = new ArrayList<>();
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
if (row == null) {
continue;
}
Data data = new Data();
data.setName(row.getCell(0).getStringCellValue());
data.setAge((int) row.getCell(1).getNumericCellValue());
dataList.add(data);
}
dataRepository.saveAll(dataList);
}
```
其中,Data 是保存数据的实体类,dataRepository 是数据访问接口。
3. 完整示例
前端页面代码:
```
<template>
<div>
<input type="file" ref="file" @change="handleFileChange">
<button @click.prevent="submit">上传</button>
</div>
</template>
<script>
import UploadComponent from 'vue-upload-component'
export default {
components: {
UploadComponent
},
data () {
return {
file: null
}
},
methods: {
handleFileChange () {
this.file = this.$refs.file.files[0]
},
submit () {
const formData = new FormData()
formData.append('file', this.file)
this.$http.post('/upload', formData)
.then(res => {
console.log(res)
})
.catch(err => {
console.log(err)
})
}
}
}
</script>
```
后端代码:
```
@RestController
public class ExcelController {
@Autowired
private DataRepository dataRepository;
@PostMapping("/upload")
public void upload(MultipartFile file) throws Exception {
Workbook workbook = WorkbookFactory.create(file.getInputStream());
Sheet sheet = workbook.getSheetAt(0);
List<Data> dataList = new ArrayList<>();
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
if (row == null) {
continue;
}
Data data = new Data();
data.setName(row.getCell(0).getStringCellValue());
data.setAge((int) row.getCell(1).getNumericCellValue());
dataList.add(data);
}
dataRepository.saveAll(dataList);
}
}
@Entity
public class Data {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Integer age;
// getter and setter
}
```
注意,需要在 application.properties 中配置数据库连接等信息。另外,需要在 Vue 项目中使用 axios 来发送请求。
这样,就完成了 Spring Boot + Vue 实现 excel 数据导入功能的示例。
阅读全文
相关推荐
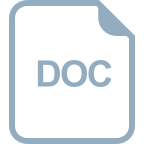
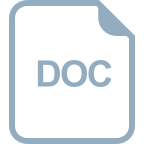
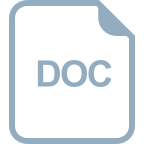

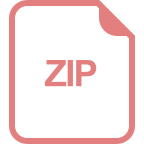

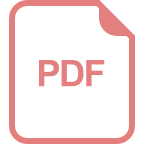
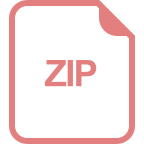


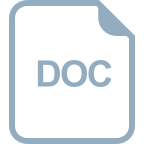
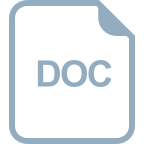
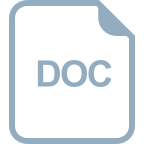
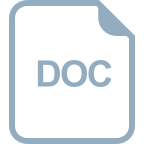
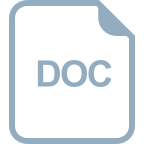
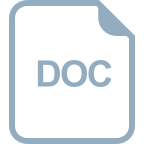
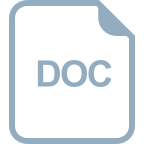
