js判断字符串中是否包含某个数据
时间: 2023-11-03 14:05:33 浏览: 185
在JavaScript中,可以使用String对象的indexOf()方法来判断一个字符串中是否包含另外一个字符。如果包含,则返回该字符在字符串中第一次出现的位置,否则返回-1。例如:
```
let str = 'hello world';
if (str.indexOf('world') !== -1) {
console.log('字符串中包含world');
} else {
console.log('字符串中不包含world');
}
```
除了indexOf()方法,还可以使用match()方法来检索字符串中是否包含某个数据。match()方法可以接受一个正则表达式作为参数,如果匹配成功,则返回一个数组,否则返回null。例如:
```
let str = 'hello world';
if (str.match(/world/)) {
console.log('字符串中包含world');
} else {
console.log('字符串中不包含world');
}
```
另外,如果需要忽略大小写,可以在正则表达式后面加上i标志,例如:
```
let str = 'hello world';
if (str.match(/WORLD/i)) {
console.log('字符串中包含world');
} else {
console.log('字符串中不包含world');
}
```
相关问题
怎么判断字符串是否包含某个值
判断字符串是否包含某个值,通常有几种常见的方法:
1. **Python** 中,你可以使用 `in` 关键字,如:
```python
str = "Hello World"
if "World" in str:
print("包含")
```
2. **JavaScript** 也类似,使用 `includes()` 方法:
```javascript
let str = "Hello World";
if (str.includes("World")) {
console.log("包含");
}
```
3. **Java** 则可以用 `contains()` 或者正则表达式 `matches()`:
```java
String str = "Hello World";
if (str.contains("World")) {
System.out.println("包含");
}
```
4. **C#** 中,使用 `Contains()` 方法:
```csharp
string str = "Hello World";
if (str.Contains("World")) {
Console.WriteLine("包含");
}
```
5. **PHP** 使用 `strpos()` 函数查找子串:
```php
$str = "Hello World";
if (strpos($str, "World") !== false) {
echo "包含";
}
```
以上都是基于字符串直接比较的方式,如果你需要处理的是数组或复杂的数据结构,可能会涉及到更复杂的搜索算法。
js 判断数据是否包含
你可以使用 JavaScript 的 `includes()` 方法来判断一个字符串或数组是否包含特定的值。
如果你想判断字符串是否包含某个子字符串,可以使用以下方法:
```javascript
const str = "Hello, world!";
const substring = "world";
if (str.includes(substring)) {
console.log("字符串包含子字符串");
} else {
console.log("字符串不包含子字符串");
}
```
如果你想判断数组是否包含某个元素,可以使用以下方法:
```javascript
const arr = [1, 2, 3, 4, 5];
const element = 3;
if (arr.includes(element)) {
console.log("数组包含元素");
} else {
console.log("数组不包含元素");
}
```
希望这能帮到你!如果有任何疑问,请随时问我。
阅读全文
相关推荐
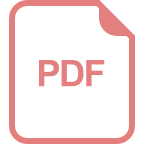
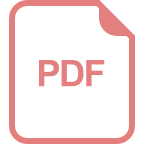
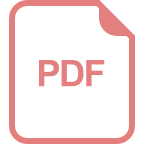













