moveToTarget(currentTag) { const $container = this.$refs.scrollContainer.$el; const $containerWidth = $container.offsetWidth; const $scrollWrapper = this.scrollWrapper; const tagList = this.$parent.$refs.tag; let firstTag = null; let lastTag = null; // find first tag and last tag if (tagList.length > 0) { firstTag = tagList[0]; lastTag = tagList[tagList.length - 1]; } if (firstTag === currentTag) { $scrollWrapper.scrollLeft = 0; } else if (lastTag === currentTag) { $scrollWrapper.scrollLeft = $scrollWrapper.scrollWidth - $containerWidth; } else { // find preTag and nextTag const currentIndex = tagList.findIndex((item) => item === currentTag); const prevTag = tagList[currentIndex - 1]; const nextTag = tagList[currentIndex + 1]; // the tag's offsetLeft after of nextTag const afterNextTagOffsetLeft = nextTag.$el.offsetLeft + nextTag.$el.offsetWidth + tagAndTagSpacing; // the tag's offsetLeft before of prevTag const beforePrevTagOffsetLeft = prevTag.$el.offsetLeft - tagAndTagSpacing; if (afterNextTagOffsetLeft > $scrollWrapper.scrollLeft + $containerWidth) { $scrollWrapper.scrollLeft = afterNextTagOffsetLeft - $containerWidth; } else if (beforePrevTagOffsetLeft < $scrollWrapper.scrollLeft) { $scrollWrapper.scrollLeft = beforePrevTagOffsetLeft; } } },
时间: 2024-02-14 13:35:26 浏览: 143
这段代码是一个移动滚动条位置的函数,其主要作用是根据当前选中的标签,移动滚动条的位置,使得选中的标签能够刚好显示在可视范围内。具体分析如下:
1. 获取滚动容器和标签列表:该函数首先获取了滚动容器和标签列表,滚动容器是通过ref属性获取的,而标签列表是通过父组件的refs属性获取的。
2. 查找第一个标签和最后一个标签:通过标签列表,函数查找到了第一个标签和最后一个标签,这两个标签的位置信息会在后面的计算中用到。
3. 判断当前标签位置:根据当前选中的标签,函数判断该标签在哪一个位置,如果是第一个标签,那么滚动条位置设置为0;如果是最后一个标签,那么滚动条位置设置为滚动容器的宽度减去滚动条的宽度;否则,需要计算标签列表中该标签前面和后面的标签位置,从而决定滚动条的位置。
4. 计算前后标签位置:为了计算前后标签的位置,函数首先通过findIndex方法查找到当前标签在标签列表中的位置,然后通过该位置计算出前一个标签和后一个标签的位置信息。
5. 移动滚动条:最后,根据计算出的前后标签位置信息,函数决定滚动条的位置,从而使得选中的标签刚好显示在可视范围内。
希望以上解析能够对您有所帮助。如果还有其他问题,请随时提出。
相关问题
document.querySelector()获取当前标签
`document.querySelector()`方法用于获取文档中与指定选择器或选择器组匹配的第一个元素。它返回一个元素对象,如果没有匹配的元素,则返回`null`。
以下是一个使用`document.querySelector()`方法获取当前标签的示例:
```javascript
const currentTag = document.querySelector(".current");
console.log(currentTag); // 输出匹配的第一个元素对象
```
在上面的示例中,我们使用选择器`.current`来获取具有`current`类的元素。如果有多个元素匹配选择器,`document.querySelector()`方法只返回第一个匹配的元素。
vue.js制作一个热门电影栏目,点击标签可以切换显示对应标签的电影
在Vue.js中创建一个热门电影栏目,你可以按照以下步骤进行:
1. **设置基础结构**:
首先,你需要创建一个HTML结构,包括一个包含多个电影列表的容器,以及一些用于切换标签的按钮。例如:
```html
<div id="movie-app">
<h2>热门电影</h2>
<ul class="movie-list" v-for="(tag, index) in movieTags" :key="index">
<li @click="showMovies(tag)">
{{ tag }}
</li>
</ul>
<div v-for="(movie, index) in displayedMovies" :key="index" :class="{ active: movie.tag === currentTag }">
<!-- 显示电影信息 -->
</div>
</div>
```
2. **Vue实例和数据绑定**:
创建一个Vue实例并定义数据,如`movieTags`数组存储所有电影标签,`displayedMovies`数组初始为空,`currentTag`用于当前显示的标签:
```javascript
new Vue({
el: '#movie-app',
data: {
movieTags: ['动作', '喜剧', '科幻'], // 样例标签
displayedMovies: [], // 存放电影列表
currentTag: ''
},
})
```
3. **计算属性和方法**:
- `computed`部分处理数据关联,比如过滤出特定标签的电影:
```javascript
computed: {
filteredMovies() {
return this.movieTags.map(tag => this.movies.find(movie => movie.tag === tag));
}
},
```
- `methods`部分添加点击标签的方法来切换电影:
```javascript
methods: {
showMovies(tag) {
this.currentTag = tag;
this.displayedMovies = this.filteredMovies; // 或者根据实际需求更新显示的电影
}
},
```
4. **模板渲染**:
使用v-if或v-show指令来根据当前标签显示相应的电影列表项:
```html
<template v-for="(movie, index) in displayedMovies">
<li :key="index">
<!-- 渲染电影信息 -->
</li>
</template>
```
5. **完整的例子**:
这只是一个基本框架,实际项目中还需要根据具体的设计和数据结构进行调整。记住要在你的项目中引入Vue.js库,并连接到数据源获取电影信息。
阅读全文
相关推荐












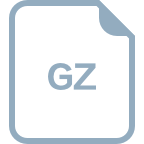

