import uvicorn from fastapi import FastAPI, UploadFile, File from io import BytesIO from PIL import Image, ImageDraw from utils.operation import YOLO def detect(onnx_path='ReqFile/yolov5n-7-k5.onnx', img=r'ReqFile/bus.jpg', show=True): ''' 检测目标,返回目标所在坐标如: {'crop': [57, 390, 207, 882], 'classes': 'person'},...] :param onnx_path:onnx模型路径 :param img:检测用的图片 :param show:是否展示 :return: ''' yolo = YOLO(onnx_path=onnx_path) # 加载yolo类 det_obj = yolo.decect(img) # 检测 # 打印检测结果 print(det_obj) # 画框框 if show: img = Image.open(img) draw = ImageDraw.Draw(img) for i in range(len(det_obj)): draw.rectangle(det_obj[i]['crop'], width=3) img.show() # 展示 return det_obj app = FastAPI() @app.get("/") def read_root(): return {"Hello": "World"} @app.post("/detect/") async def create_upload_file(file: UploadFile = File(...)): contents = await file.read() # 接收浏览器上传的图片 im1 = BytesIO(contents) # 将数据流转换成二进制文件存在内存中 # 返回结果 return detect(onnx_path='ReqFile/best-0206.onnx', img=im1, show=False) # 启动项目 if __name__ == "__main__": uvicorn.run(app='main:app', port=8000, host='0.0.0.0', reload=True)
时间: 2024-04-28 18:21:02 浏览: 12
这段代码使用 FastAPI 框架搭建了一个接口,可以接收浏览器上传的图片,调用 `detect()` 函数进行目标检测,最后返回检测结果。其中使用了 YOLO 模型进行目标检测,`detect()` 函数接收 ONNX 模型路径和图片,返回目标在图片中的位置和类别。`create_upload_file()` 函数接收上传的图片,将其转换成二进制文件存在内存中,调用 `detect()` 函数进行目标检测,并返回结果。最后使用 Uvicorn 启动项目。
相关问题
ModuleNotFoundError: No module named 'fastapi.middleware.multipart'
这个错误通常是因为你的代码中使用了 FastAPI 版本低于 0.60.0,而 `fastapi.middleware.multipart` 模块是在 0.60.0 版本中添加的。你可以升级 FastAPI 到最新版本,使用以下命令:
```
pip install fastapi --upgrade
```
如果你需要使用旧版本的 FastAPI,你可以使用 `python-multipart` 模块来处理 `multipart/form-data` 数据格式,而不需要使用 `fastapi.middleware.multipart` 模块。你可以使用以下代码来处理 `multipart/form-data` 数据格式:
```python
from fastapi import FastAPI, Form, File, UploadFile
from fastapi.param_functions import Depends
from fastapi.security import OAuth2PasswordRequestForm
from fastapi.middleware.cors import CORSMiddleware
from fastapi.responses import JSONResponse
from fastapi.exceptions import RequestValidationError
app = FastAPI()
# 添加 CORS 中间件
origins = [
"http://localhost",
"http://localhost:8000",
"http://localhost:8080",
"https://localhost",
"https://localhost:8000",
"https://localhost:8080",
]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
# 定义路由和处理函数
@app.post("/uploadfile/")
async def create_upload_file(file: bytes = File(...)):
return {"file_size": len(file)}
@app.post("/form/")
async def create_form_file(file: bytes = Form(...)):
return {"file_size": len(file)}
@app.post("/uploadfiles/")
async def create_upload_files(files: list = File(...)):
return {"file_sizes": [len(file) for file in files]}
@app.post("/login/")
async def login(form_data: OAuth2PasswordRequestForm = Depends()):
return {"username": form_data.username}
```
在上面的代码中,我们使用 `File` 和 `Form` 参数来处理 `multipart/form-data` 数据格式。
flutter MultipartFile.fromFile
`MultipartFile.fromFile` 是 Flutter 中的一个方法,用于创建一个 `MultipartFile` 对象来表示一个文件上传的数据。这个方法需要一个文件路径作为参数,它会读取指定路径的文件,并将该文件的内容转换为一个 `MultipartFile` 对象。
下面是一个示例代码,演示如何使用 `MultipartFile.fromFile` 方法:
```dart
import 'dart:io';
import 'package:http/http.dart' as http;
void uploadFile(String filePath) async {
var file = File(filePath);
var stream = http.ByteStream(file.openRead());
var length = await file.length();
var uri = Uri.parse('https://example.com/upload'); // 替换为实际的上传地址
var request = http.MultipartRequest('POST', uri);
var multipartFile = http.MultipartFile('file', stream, length,
filename: file.path.split('/').last);
request.files.add(multipartFile);
var response = await request.send();
if (response.statusCode == 200) {
print('File uploaded successfully');
} else {
print('Error uploading file');
}
}
```
在上面的例子中,我们首先创建了一个 `File` 对象来表示要上传的文件,然后使用 `file.openRead()` 方法获取文件的字节流,并使用 `file.length()` 方法获取文件的长度。
接下来,我们创建一个 `Uri` 对象来表示上传地址,并使用 `http.MultipartRequest` 创建一个 `http.Request` 对象。然后,我们使用 `MultipartFile.fromFile` 方法将文件转换为 `MultipartFile` 对象,并将其添加到请求中。
最后,我们使用 `request.send()` 方法发送请求,并根据响应的状态码判断上传是否成功。
请注意,上述示例中的上传地址和文件参数仅用作示例,请根据实际情况进行修改。
相关推荐
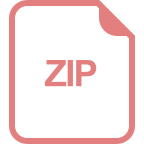
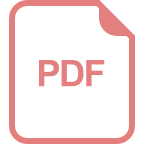
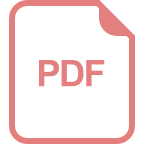












