GNN 代码实现图编码
时间: 2023-09-17 08:12:46 浏览: 57
GNN(Graph Neural Network,图神经网络)是一种可以对图结构数据进行学习和推理的神经网络模型。GNN 的主要思想是将图中的节点和边作为神经网络的输入,通过迭代更新节点的隐藏状态,最终得到图的表示。下面是一个简单的 GNN 代码实现示例:
首先,我们需要定义一个 Graph 类来表示图结构,包括节点数、边数、邻接矩阵等信息:
```python
class Graph():
def __init__(self, num_nodes, num_edges, adjacency_matrix):
self.num_nodes = num_nodes
self.num_edges = num_edges
self.adjacency_matrix = adjacency_matrix
```
接着,我们可以定义一个 GNN 模型类,其中包含了节点嵌入函数和 GNN 更新函数:
```python
class GNN():
def __init__(self, input_dim, hidden_dim, output_dim):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.node_embedding = nn.Linear(input_dim, hidden_dim)
self.gnn_update = nn.Linear(hidden_dim, hidden_dim)
self.output_layer = nn.Linear(hidden_dim, output_dim)
def forward(self, graph):
hidden_states = self.node_embedding(graph.adjacency_matrix)
for i in range(num_iterations):
hidden_states = self.gnn_update(torch.matmul(graph.adjacency_matrix, hidden_states))
output = self.output_layer(torch.mean(hidden_states, dim=0))
return output
```
在 forward 函数中,首先对节点进行嵌入操作,然后进行 num_iterations 次 GNN 更新操作,最后通过输出层得到图的表示。其中,nn.Linear 表示全连接层,torch.matmul 表示矩阵乘法,torch.mean 表示对张量进行平均操作。
最后,我们可以使用上述定义的 Graph 类和 GNN 模型类对一个示例图进行编码:
```python
# 构建示例图
num_nodes = 5
num_edges = 6
adjacency_matrix = torch.Tensor([[0, 1, 1, 0, 0],
[1, 0, 0, 1, 0],
[1, 0, 0, 1, 1],
[0, 1, 1, 0, 1],
[0, 0, 1, 1, 0]])
graph = Graph(num_nodes, num_edges, adjacency_matrix)
# 构建 GNN 模型
input_dim = 1
hidden_dim = 16
output_dim = 1
gnn_model = GNN(input_dim, hidden_dim, output_dim)
# 对示例图进行编码
gnn_output = gnn_model(graph)
```
注意,上述代码仅为示例,实际应用中可能需要根据具体需求对 GNN 模型进行进一步改进和优化。
相关推荐
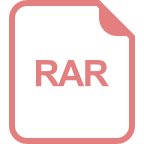
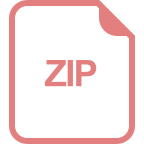


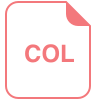











