优化这段代码的运行时间#include<stdio.h> #include<stdlib.h> typedef struct node* DNode; struct node { int data; DNode prior; //前面数据地址 DNode next; //后面数据地址 }; //创建双向链表 void CreatNode(DNode *head) { DNode s; //新节点指针 char e; (*head) = (DNode)malloc(sizeof(struct node));//头结点 (*head)->prior = (*head); //初始头结点的前驱和后驱都指向自己 (*head)->next = (*head); printf("输入数据\n"); scanf("%c", &e); while (e!='\n') { s = (DNode)malloc(sizeof(struct node)); //新节点分配空间 s->data = e; s->prior = (*head); //新节点的prior连前一个结点 s->next = (*head)->next; //新节点的next连后边结点 (*head)->next->prior = s; //后一个结点的prior连新结点 (*head)->next = s; //新节点前面的next连新结点 scanf("%c", &e); } } //向后遍历输出 void PrintList1(DNode L) { DNode p; p = L; p = p->next; while (p != L) { printf("%c", p->data); p = p->next; } printf("\n"); } //向前遍历输出 void PrintList2(DNode L) { DNode p; p = L->prior; while (p != L) { printf("%c", p->data); p = p->prior; } printf("\n"); } //查找第i处数据的地址 DNode FindPosition(DNode L,int i) { int j = 0; DNode p = L; while (p->next != L&&j < i) { p = p->next; j++; } return p; } //插入 void InsertList(DNode L) { DNode s,p; //s为新结点 p为新节点前一个结点 int i; char e; printf("在第几处插入:\n"); scanf("%d", &i); getchar(); printf("插入什么数据:\n"); scanf("%c", &e); p = FindPosition(L, i-1); //新节点前一个结点地址 s = (DNode)malloc(sizeof(struct node));//申请新节点空间 s->data = e; s->prior = p; //新节点的prior连上前一个结点 s->next = p->next; //新节点的next连上后一个结点 p->next->prior = s; //新节点后的结点的prior连上新结点 p->next = s; //新节点前的结点的next连上新结点 } //删除 void DeleteList(DNode L){ DNode s,p; //s为新结点 p为要删除的结点 int i; printf("删除第几处的数据:\n"); scanf("%d", &i); p = FindPosition(L, i); //要删除结点的地址 p->prior->next = p->next; //要删除的结点的前一个结点的next,连上要删结点后的结点 p->next->prior = p->prior;//要删除结点的后一个结点的prior,连上要删结点的前一个结点 free(p); } int main() { DNode list; CreatNode(&list); //PrintList1(list); PrintList2(list); InsertList(list); PrintList2(list); DeleteList(list); PrintList2(list); }
时间: 2023-05-17 14:04:41 浏览: 81
要优化这段代码的运行时间,可以考虑以下几个方面:
1. 减少循环次数:如果代码中有循环语句,可以尝试减少循环次数,比如使用更高效的算法或数据结构来代替循环。
2. 减少重复计算:如果代码中有重复计算的部分,可以尝试将其缓存起来,避免重复计算。
3. 减少函数调用:函数调用会带来一定的开销,如果代码中有频繁的函数调用,可以尝试将其合并或优化。
4. 使用并行计算:如果代码中有可以并行计算的部分,可以尝试使用多线程或分布式计算来加速运行。
5. 优化内存使用:如果代码中有大量的内存分配和释放操作,可以尝试优化内存使用,比如使用对象池或缓存来避免频繁的内存分配和释放。
6. 使用更高效的数据结构和算法:如果代码中使用的数据结构和算法不够高效,可以尝试使用更高效的数据结构和算法来代替。
相关问题
#include <stdio.h> #include <stdlib.h> typedef int ElemType; typedef struct LNode { ElemType da
`#include <stdio.h>`和`#include <stdlib.h>`是C语言中的两个头文件。`<stdio.h>`是标准输入输出头文件,提供了一些用于输入输出的函数和宏定义。`<stdlib.h>`是标准库头文件,提供了一些常用的函数和宏定义,如内存分配、随机数生成等。
在你的代码中,`typedef int ElemType;`定义了一个类型别名,将`int`类型命名为`ElemType`。
`typedef struct LNode`定义了一个结构体类型`LNode`,结构体是一种自定义的数据类型,可以包含多个不同类型的成员变量。在这里,`LNode`结构体中的成员变量还没有完整定义,因此代码截断了。
#include <stdio.h> #include <stdlib.h> #define MAXSIZE 100 typedef int ElemType; typedef struct
这段代码是C语言中的头文件引用和一些定义的示例。其中,`#include <stdio.h>`和`#include <stdlib.h>`是用来引入标准库函数的头文件。`stdio.h`包含了输入输出相关的函数,如`printf`和`scanf`;`stdlib.h`包含了一些常用的函数,如内存分配函数`malloc`和随机数生成函数`rand`。
`#define MAXSIZE 100`是一个宏定义,用来定义一个常量`MAXSIZE`,它的值为100。
`typedef int ElemType;`是用来给`int`类型起一个别名`ElemType`,方便在代码中使用。
至于代码中的结构体部分,由于你没有提供完整的代码,所以无法给出具体的解释。但一般来说,结构体用于定义一种自定义的数据类型,可以包含多个不同类型的成员变量。
相关推荐
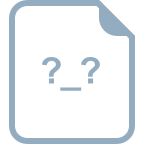
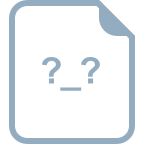













