在Eclipse中创建了Dynamic Web Project项目,项目名称为login1,请编写一个简单的学生信息管理系统需要连接MySQL数据库,请把哪个文件和创在哪和哪个文件所有代码发给我
时间: 2024-02-06 11:09:36 浏览: 23
你需要创建以下文件和代码:
1. 在WebContent文件夹下创建index.jsp文件,用于展示学生信息管理系统的主页面。
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生信息管理系统</title>
</head>
<body>
<h1>学生信息管理系统</h1>
<a href="login.jsp">登录</a>
</body>
</html>
```
2. 在WebContent文件夹下创建login.jsp文件,用于用户登录。
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>登录</title>
</head>
<body>
<h1>登录</h1>
<form action="login" method="post">
<label for="username">用户名:</label>
<input type="text" name="username"><br>
<label for="password">密码:</label>
<input type="password" name="password"><br>
<input type="submit" value="登录">
</form>
</body>
</html>
```
3. 在src文件夹下创建com.login1.dao包,用于存放数据库操作相关的类。在该包下创建StudentDao类,用于操作学生信息表。
```java
package com.login1.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.login1.entity.Student;
public class StudentDao {
private Connection conn;
private void getConnection() throws SQLException {
String url = "jdbc:mysql://localhost:3306/login1";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
}
public List<Student> findAll() throws SQLException {
List<Student> list = new ArrayList<Student>();
getConnection();
String sql = "SELECT * FROM student";
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
Student student = new Student();
student.setId(rs.getInt("id"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
student.setMajor(rs.getString("major"));
list.add(student);
}
rs.close();
pstmt.close();
conn.close();
return list;
}
public Student findByName(String name) throws SQLException {
Student student = null;
getConnection();
String sql = "SELECT * FROM student WHERE name=?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, name);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
student = new Student();
student.setId(rs.getInt("id"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
student.setMajor(rs.getString("major"));
}
rs.close();
pstmt.close();
conn.close();
return student;
}
}
```
4. 在src文件夹下创建com.login1.entity包,用于存放实体类。在该包下创建Student类,用于封装学生信息。
```java
package com.login1.entity;
public class Student {
private int id;
private String name;
private int age;
private String major;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getMajor() {
return major;
}
public void setMajor(String major) {
this.major = major;
}
}
```
5. 在src文件夹下创建com.login1.servlet包,用于存放Servlet类。在该包下创建LoginServlet类,用于处理用户登录请求。
```java
package com.login1.servlet;
import java.io.IOException;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.login1.dao.StudentDao;
import com.login1.entity.Student;
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String username = request.getParameter("username");
String password = request.getParameter("password");
StudentDao studentDao = new StudentDao();
try {
Student student = studentDao.findByName(username);
if (student == null) {
request.setAttribute("msg", "用户名不存在");
request.getRequestDispatcher("/login.jsp").forward(request, response);
} else if (!password.equals("123456")) {
request.setAttribute("msg", "密码错误");
request.getRequestDispatcher("/login.jsp").forward(request, response);
} else {
request.getSession().setAttribute("student", student);
response.sendRedirect("list.jsp");
}
} catch (SQLException e) {
e.printStackTrace();
request.setAttribute("msg", "系统错误");
request.getRequestDispatcher("/login.jsp").forward(request, response);
}
}
}
```
6. 在WebContent文件夹下创建list.jsp文件,用于展示学生信息列表。
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生信息列表</title>
</head>
<body>
<h1>学生信息列表</h1>
<table border="1">
<tr>
<th>编号</th>
<th>姓名</th>
<th>年龄</th>
<th>专业</th>
</tr>
<c:forEach var="student" items="${students}">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>${student.age}</td>
<td>${student.major}</td>
</tr>
</c:forEach>
</table>
<p><a href="logout.jsp">退出登录</a></p>
</body>
</html>
```
7. 在WebContent文件夹下创建logout.jsp文件,用于处理用户退出登录请求。
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>退出登录</title>
</head>
<body>
<%
request.getSession().invalidate();
response.sendRedirect("index.jsp");
%>
</body>
</html>
```
以上就是一个简单的学生信息管理系统的所有代码,其中需要连接MySQL数据库的部分在StudentDao类中实现。你需要自行创建MySQL数据库,并在getConnection()方法中修改url、user和password变量的值以连接到你的数据库。
相关推荐
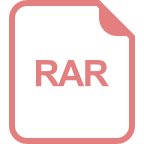
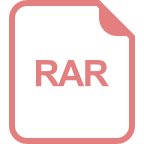
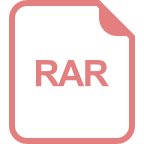














