在python的keras中对一幅64*64二值图像置乱的水印图像进行标准化处理后,与resnet50输出的1*1*4096的向量进行异或操作,生成一幅新的64*64的灰度图
时间: 2023-12-05 11:04:15 浏览: 27
可以按照以下步骤进行操作:
1. 读取原始二值图像和水印图像,将它们转换为keras中的标准数据格式,例如numpy数组或keras张量。
2. 对二值图像和水印图像进行标准化处理,将它们的像素值缩放到[0,1]之间,可以使用keras中的preprocessing模块中的函数进行标准化处理。
3. 将标准化后的水印图像与resnet50模型输出的1x1x4096向量进行异或操作,生成一幅新的1x4096的向量。
4. 将新的1x4096向量重新调整为64x64的灰度图像,可以使用numpy中的reshape函数进行重构。
5. 将生成的新灰度图像保存到文件中,以供后续使用。
下面是一个简单的代码示例:
```python
from keras.preprocessing import image
from keras.applications.resnet50 import ResNet50
import numpy as np
import cv2
# 读取二值图像和水印图像
img_path = 'binary_image.png'
watermark_path = 'watermark.png'
img = image.load_img(img_path, target_size=(64, 64), color_mode='grayscale')
watermark = image.load_img(watermark_path, target_size=(64, 64), color_mode='grayscale')
# 将图像转为numpy数组,并进行标准化处理
img = image.img_to_array(img)
img /= 255.0
watermark = image.img_to_array(watermark)
watermark /= 255.0
# 加载resnet50模型,并进行预测
model = ResNet50(weights='imagenet', include_top=False, pooling='avg')
watermark_vector = model.predict(np.array([watermark]))
# 将水印向量与原始二值图像进行异或操作,生成新的向量
new_vector = watermark_vector ^ img.reshape(1, 4096)
# 将新的向量重新调整为64x64的灰度图像
new_img = new_vector.reshape(64, 64)
# 将新的灰度图像保存到文件中
cv2.imwrite('new_image.png', new_img)
```
需要注意的是,由于图像是灰度图像,所以在读取和保存时需要使用color_mode='grayscale'选项。另外,由于resnet50模型的输出是1x1x4096的向量,因此需要使用reshape函数将其调整为1维的4096向量。
相关推荐
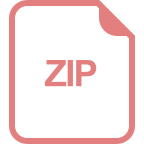
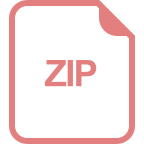














