vue 滚动公告
时间: 2023-06-29 15:05:36 浏览: 105
可以使用 Vue.js 中的 `vue-marquee-text-component` 组件来实现滚动公告。
首先,需要安装这个组件。可以使用 npm 或者 yarn 安装:
```
npm install vue-marquee-text-component
```
或者
```
yarn add vue-marquee-text-component
```
接下来,在 Vue 组件中引入这个组件,并在模板中使用它:
```html
<template>
<div class="scroll-notice">
<marquee-text :speed="50">
这里是滚动公告内容
</marquee-text>
</div>
</template>
<script>
import MarqueeText from 'vue-marquee-text-component'
export default {
components: {
MarqueeText
}
}
</script>
```
这样就可以实现一个简单的滚动公告了。你也可以自定义滚动速度、滚动方向等属性。具体使用方法可以参考 `vue-marquee-text-component` 的文档。
相关问题
vue滚动公告
要在Vue中实现滚动公告,可以使用CSS的动画,结合Vue的生命周期函数和定时器,来实现循环滚动的效果。以下是一个简单的示例代码:
```
<template>
<div class="announcement">
<div class="content" ref="content">{{ currentAnnouncement }}</div>
</div>
</template>
<script>
export default {
data() {
return {
announcements: ['Announcement 1', 'Announcement 2', 'Announcement 3'],
currentIndex: 0,
animationDuration: 3000 // 滚动间隔时间,单位为毫秒
}
},
computed: {
currentAnnouncement() {
return this.announcements[this.currentIndex]
}
},
mounted() {
this.startAnimation()
},
beforeDestroy() {
clearInterval(this.timer)
},
methods: {
startAnimation() {
this.timer = setInterval(() => {
this.currentIndex = (this.currentIndex + 1) % this.announcements.length
this.$refs.content.style.animation = `scroll ${this.animationDuration / 1000}s linear`
setTimeout(() => {
this.$refs.content.style.animation = ''
}, this.animationDuration)
}, this.animationDuration)
}
}
}
</script>
<style>
.announcement {
overflow: hidden;
height: 20px; /* 公告栏的高度 */
}
.content {
display: inline-block;
white-space: nowrap;
animation-timing-function: linear;
}
@keyframes scroll {
0% {
transform: translateX(0);
}
100% {
transform: translateX(calc(-100% + 100vw)); /* 计算滚动距离 */
}
}
</style>
```
这个示例中,我们将公告栏的高度设置为20px,并将文字内容放在一个inline-block元素中。然后,我们在CSS中定义了一个名为scroll的动画,让文字内容沿着X轴方向滚动。在Vue组件的mounted生命周期函数中,我们调用了startAnimation方法,该方法使用setInterval定时器来控制滚动的节奏。在每次滚动之前,我们设置了animation属性,来触发CSS动画。当动画结束后,我们使用setTimeout方法来清除animation属性,让内容回到原位。
vue 公告滚动效果
Vue 中公告滚动效果通常是指在一个滚动区域(如轮播图、滚动列表或消息面板)展示动态更新的内容,比如系统通知或新闻。实现这种效果的一种常见做法是使用 Vue 的响应式特性结合 CSS 或动画库(如 Vuetify 的 `v-loading` 或 Vanilla JavaScript 的 `Intersection Observer API`)。步骤大致如下:
1. **创建组件**:定义一个公告组件,包含消息列表和滚动功能。
```html
<template>
<div class="scrolling-container">
<ul ref="scrollList" v-for="announcement in announcements" :key="announcement.id">
<!-- 每条公告的模板 -->
<li>{{ announcement.content }}</li>
</ul>
<button @click="loadMore">加载更多</button>
</div>
</template>
<script>
export default {
data() {
return {
announcements: [],
isScrolling: false,
hasMore: true, // 初始状态表示还有更多内容
};
},
methods: {
loadMore() {
if (this.hasMore) {
this.$axios.get('api/announcements') // 使用 API 获取更多数据
.then(response => {
this.announcements.push(...response.data);
this.isScrolling = true;
setTimeout(() => { // 等待滚动完成后再停止
this.isScrolling = false;
}, 500); // 假设动画延迟500ms
});
}
},
},
};
</script>
```
2. **CSS样式**:添加 CSS 来实现滚动效果和加载提示。例如,可以设置滚动监听器并在滚动到容器底部时触发 `loadMore` 方法。
```css
.scrolling-container {
overflow-y: auto; /* 自动滚动 */
}
.load-more {
display: none;
position: fixed; bottom: 20px;
width: 80%;
text-align: center;
}
.is-scrolling & {
display: block;
}
```
阅读全文
相关推荐
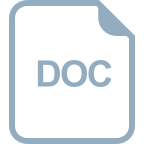
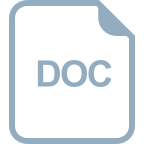
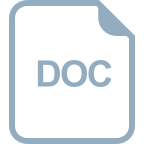
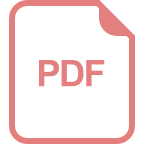
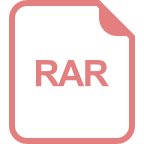
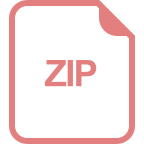










