练习JDBC,本地mysql创建数据库ie2121,创建表格student,包括字段id, name, phone, score. 通过jdbc,插入一条数据并查找,然后修改score,再查查询修改过的结果。
时间: 2024-02-24 22:54:42 浏览: 91
好的,我来为您提供相关的 JDBC 代码示例。
首先,您需要下载并导入 MySQL Connector/J 驱动程序。然后,您可以使用以下代码创建数据库和表:
```java
import java.sql.*;
public class CreateDatabaseAndTable {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
// 注册 JDBC 驱动
Class.forName("com.mysql.cj.jdbc.Driver");
// 打开链接
System.out.println("连接数据库...");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/?serverTimezone=UTC", "root", "password");
// 创建数据库
System.out.println("创建数据库...");
stmt = conn.createStatement();
String sql = "CREATE DATABASE ie2121";
stmt.executeUpdate(sql);
System.out.println("数据库已创建!");
// 选择数据库
System.out.println("选择数据库...");
stmt.close();
conn.close();
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/ie2121?serverTimezone=UTC", "root", "password");
System.out.println("数据库已选择!");
// 创建表格
System.out.println("创建表格...");
stmt = conn.createStatement();
sql = "CREATE TABLE student " +
"(id INT NOT NULL AUTO_INCREMENT, " +
" name VARCHAR(255), " +
" phone VARCHAR(255), " +
" score INT, " +
" PRIMARY KEY ( id ))";
stmt.executeUpdate(sql);
System.out.println("表格已创建!");
} catch (SQLException se) {
// 处理 JDBC 错误
se.printStackTrace();
} catch (Exception e) {
// 处理 Class.forName 错误
e.printStackTrace();
} finally {
// 关闭资源
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
} // 什么都不做
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
}
}
```
接下来,您可以使用以下代码插入一条数据并查询它:
```java
import java.sql.*;
public class InsertAndSelect {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement pstmt = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 注册 JDBC 驱动
Class.forName("com.mysql.cj.jdbc.Driver");
// 打开链接
System.out.println("连接数据库...");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/ie2121?serverTimezone=UTC", "root", "password");
// 插入数据
System.out.println("插入数据...");
String sql = "INSERT INTO student (name, phone, score) VALUES (?, ?, ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "Tom");
pstmt.setString(2, "123456789");
pstmt.setInt(3, 90);
pstmt.executeUpdate();
// 查询数据
System.out.println("查询数据...");
stmt = conn.createStatement();
sql = "SELECT * FROM student";
rs = stmt.executeQuery(sql);
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String phone = rs.getString("phone");
int score = rs.getInt("score");
System.out.println("ID: " + id + ", Name: " + name + ", Phone: " + phone + ", Score: " + score);
}
} catch (SQLException se) {
// 处理 JDBC 错误
se.printStackTrace();
} catch (Exception e) {
// 处理 Class.forName 错误
e.printStackTrace();
} finally {
// 关闭资源
try {
if (rs != null) rs.close();
} catch (SQLException se1) {
} // 什么都不做
try {
if (pstmt != null) pstmt.close();
} catch (SQLException se2) {
} // 什么都不做
try {
if (stmt != null) stmt.close();
} catch (SQLException se3) {
} // 什么都不做
try {
if (conn != null) conn.close();
} catch (SQLException se4) {
se4.printStackTrace();
}
}
}
}
```
最后,您可以使用以下代码修改分数并查询它:
```java
import java.sql.*;
public class UpdateAndSelect {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement pstmt = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 注册 JDBC 驱动
Class.forName("com.mysql.cj.jdbc.Driver");
// 打开链接
System.out.println("连接数据库...");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/ie2121?serverTimezone=UTC", "root", "password");
// 修改分数
System.out.println("修改分数...");
String sql = "UPDATE student SET score = ? WHERE name = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, 80);
pstmt.setString(2, "Tom");
pstmt.executeUpdate();
// 查询数据
System.out.println("查询数据...");
stmt = conn.createStatement();
sql = "SELECT * FROM student";
rs = stmt.executeQuery(sql);
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String phone = rs.getString("phone");
int score = rs.getInt("score");
System.out.println("ID: " + id + ", Name: " + name + ", Phone: " + phone + ", Score: " + score);
}
} catch (SQLException se) {
// 处理 JDBC 错误
se.printStackTrace();
} catch (Exception e) {
// 处理 Class.forName 错误
e.printStackTrace();
} finally {
// 关闭资源
try {
if (rs != null) rs.close();
} catch (SQLException se1) {
} // 什么都不做
try {
if (pstmt != null) pstmt.close();
} catch (SQLException se2) {
} // 什么都不做
try {
if (stmt != null) stmt.close();
} catch (SQLException se3) {
} // 什么都不做
try {
if (conn != null) conn.close();
} catch (SQLException se4) {
se4.printStackTrace();
}
}
}
}
```
希望这些代码能够帮助您练习 JDBC。
阅读全文
相关推荐


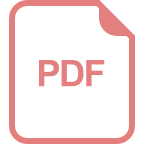
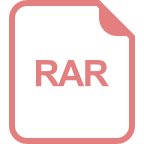
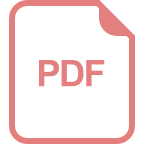
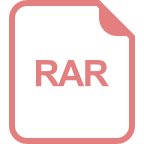
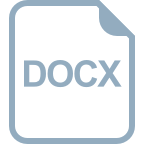
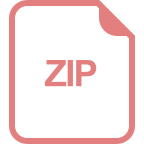
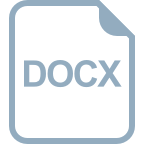
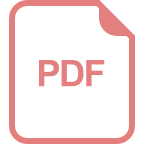
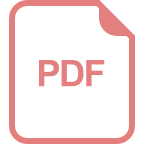
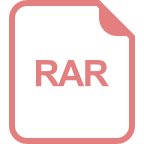
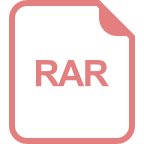
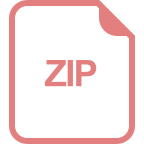
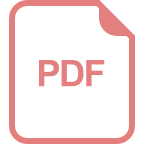