统计文件【hamlet.txt】中小写字母a-z的字符分布,即出现a-z字符的数量,并输出结果。同时请输出文件一共包含的字符数量。Python实现
时间: 2024-02-24 19:55:20 浏览: 32
可以使用 Python 的内置模块 `collections` 中的 `Counter` 类来统计字符分布,代码如下:
```python
import collections
# 打开文件
with open("hamlet.txt", "r", encoding="UTF-8") as f:
# 读取文件内容
content = f.read()
# 统计字符分布
counter = collections.Counter(content.lower())
# 输出结果
for c in "abcdefghijklmnopqrstuvwxyz":
print(f"{c}: {counter.get(c, 0)}")
# 输出字符总数
print(f"Total: {len(content)}")
```
上面代码中,首先使用 `open` 函数打开文件,并通过 `read` 方法读取文件内容。然后,使用 `collections.Counter` 统计字符分布,并使用 `get` 方法获取指定字符的数量,如果该字符没有出现过,则返回 0。最后,使用 `len` 函数获取文件总字符数并输出。
相关问题
统计文件【hamlet.txt】中小写字母a-z的字符分布,即出现a-z字符的数量,并输出结果。同时请输出文件一共包含的字符数量
好的,可以使用Python的内置模块collections来实现。以下是代码实现:
```python
import collections
with open('hamlet.txt', 'r', encoding='UTF-8') as f:
lines = f.readlines()
# 将所有行拼接成一个字符串
text = ''.join(lines)
# 统计小写字母a-z的出现次数
counter = collections.Counter(c for c in text.lower() if 'a' <= c <= 'z')
# 输出字符分布
for c in 'abcdefghijklmnopqrstuvwxyz':
print(f"{c}: {counter[c]}")
# 输出文件包含的字符数量
print(f"文件包含的字符数量:{len(text)}")
```
注意,需要将文件名替换为实际的文件名。输出的结果会按照字母表顺序逐行显示每个字母的出现次数,最后一行输出文件总字符数。
4、统计文件【hamlet.txt】中小写字母a-z的字符分布,即出现a-z字符的数量,并输出结果。同时请输出文件一共包含的字符数量。
以下是统计文件中小写字母a-z的字符分布并输出结果的Python代码:
```python
with open('hamlet.txt', 'r') as f:
content = f.read()
char_count = {}
for char in content:
if char.islower():
char_count[char] = char_count.get(char, 0) + 1
total_count = sum(char_count.values())
print("小写字母a-z的字符分布:")
for char, count in char_count.items():
print(char, ": ", count)
print("文件一共包含的字符数量:", total_count)
```
其中,将文件路径替换成实际的文件路径即可运行。
阅读全文
相关推荐


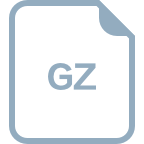













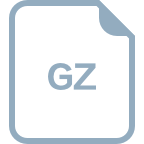
