C#实现当前ssh远程windows运行某个java命令,如果该命令15分钟内仍未结束则杀死该进程并执行下一条java命令
时间: 2024-10-21 12:16:02 浏览: 38
在C#中,你可以使用`System.Diagnostics.Process`类来控制远程Windows机器上的进程,并结合定时任务来实现这个需求。这里是一个简化的示例,展示了如何通过SSH连接执行Java命令,设置超时并监控进程是否完成:
首先,你需要安装SSH.NET库来处理SSH连接,可以参考其官方文档:https://sshnet.codeplex.com/
```csharp
using System;
using System.Diagnostics;
using SSH.NET;
public class RemoteCommandExecutor
{
private const string SshCommandFormat = @"plink -i {privateKeyPath} user@remoteHost 'cmd /c {javaCommand}'";
public void ExecuteJavaCommands(string privateKeyPath, string remoteHost, List<string> commands, TimeSpan commandTimeout)
{
using (var client = new SshClient())
{
// 设置SSH客户端配置
client.HostName = remoteHost;
client.Username = "user"; // 远程机器用户名
client.IdentityFile = privateKeyPath; // 私钥文件路径
foreach (string command in commands)
{
ProcessStartInfo psi = new ProcessStartInfo();
psi.FileName = "cmd.exe";
psi.Arguments = $"/c \"{command}\""; // Java命令字符串
var process = client.StartShell(psi); // 使用SSH启动命令
var timeoutTimer = new Timer(commandTimeout.TotalMilliseconds);
timeoutTimer.Elapsed += (_, e) =>
{
if (!process.HasExited) // 判断进程是否已退出
{
process.Kill(); // 如果超时未结束,强制终止进程
}
else
{
timeoutTimer.Stop(); // 否则,关闭超时计时器
}
};
timeoutTimer.Start();
process.WaitForExit(); // 等待命令完成或超时
}
}
}
}
// 调用示例:
string privateKeyPath = @"path/to/private/key.pem";
string remoteHost = "your.remote.host";
List<string> javaCommands = new List<string> { "java -jar your-jar.jar arg1 arg2", /*更多命令*/ };
RemoteCommandExecutor executor = new RemoteCommandExecutor();
executor.ExecuteJavaCommands(privateKeyPath, remoteHost, javaCommands, TimeSpan.FromMinutes(15));
```
阅读全文
相关推荐
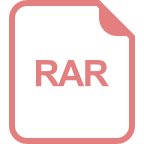
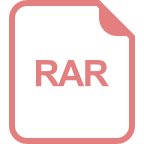
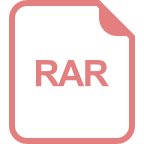
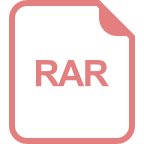
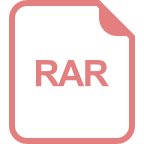
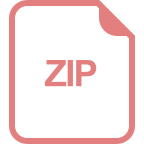
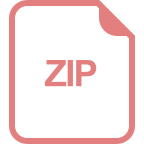
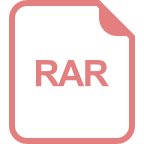
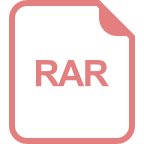
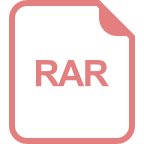
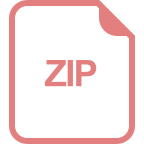
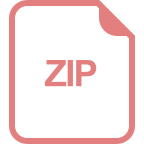
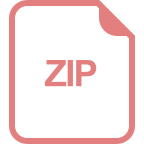
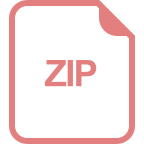
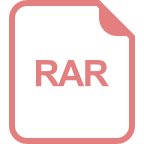
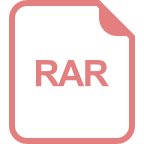
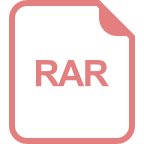
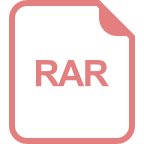