使用Python的pytorch完成两张照片的神经风格转换,其中内容照片路径为'D:\\Python\\results\\1\\dancing.jpg',风格照片路径为'D:\\Python\\results\\1\\picasso.jpg'
时间: 2024-03-28 20:35:06 浏览: 19
使用Python的PyTorch完成两张照片的神经风格转换可以通过以下步骤实现:
1. 导入所需的库和模块:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import transforms, models
from PIL import Image
```
2. 定义风格转换网络:
```python
class StyleTransferNet(nn.Module):
def __init__(self):
super(StyleTransferNet, self).__init__()
# 定义网络结构,可以使用预训练的VGG模型作为基础
# 可以根据需要自定义网络结构和层数
self.model = models.vgg19(pretrained=True).features[:23]
def forward(self, x):
return self.model(x)
```
3. 加载内容照片和风格照片:
```python
content_path = 'D:\\Python\\results\\1\\dancing.jpg'
style_path = 'D:\\Python\\results\\1\\picasso.jpg'
content_image = Image.open(content_path)
style_image = Image.open(style_path)
```
4. 对图片进行预处理:
```python
preprocess = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(256),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
content_tensor = preprocess(content_image).unsqueeze(0)
style_tensor = preprocess(style_image).unsqueeze(0)
```
5. 创建风格转换网络实例和目标图像的张量:
```python
net = StyleTransferNet()
target_tensor = content_tensor.clone().requires_grad_(True)
```
6. 定义损失函数和优化器:
```python
criterion = nn.MSELoss()
optimizer = optim.Adam([target_tensor], lr=0.01)
```
7. 进行风格转换的迭代训练:
```python
num_epochs = 1000
for epoch in range(num_epochs):
optimizer.zero_grad()
output = net(target_tensor)
content_loss = criterion(output, net(content_tensor))
style_loss = criterion(gram_matrix(output), gram_matrix(net(style_tensor)))
total_loss = content_loss + style_loss
total_loss.backward()
optimizer.step()
```
8. 保存生成的图像:
```python
output_image = target_tensor.detach().squeeze(0)
output_image = output_image.permute(1, 2, 0).numpy()
output_image = output_image * [0.229, 0.224, 0.225] + [0.485, 0.456, 0.406]
output_image = (output_image * 255).clip(0, 255).astype('uint8')
output_path = 'D:\\Python\\results\\1\\output.jpg'
Image.fromarray(output_image).save(output_path)
```
以上是使用Python的PyTorch完成两张照片的神经风格转换的基本步骤。你可以根据需要进行调整和优化。
相关推荐
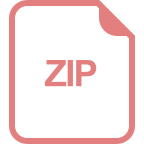
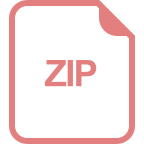
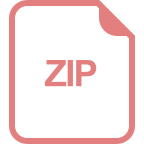














