请用C语言写一个栈堆的代码
时间: 2024-05-03 08:21:35 浏览: 55
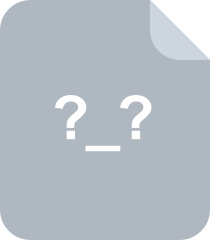
c语言的栈源代码
栈是一种后进先出的数据结构,可以使用数组或链表来实现。以下是使用数组实现栈的代码:
```c
#include <stdio.h>
#define MAX_SIZE 10
int stack[MAX_SIZE];
int top = -1;
int isFull() {
if (top == MAX_SIZE - 1) {
return 1;
} else {
return 0;
}
}
int isEmpty() {
if (top == -1) {
return 1;
} else {
return 0;
}
}
void push(int value) {
if (isFull()) {
printf("Stack is full\n");
} else {
top++;
stack[top] = value;
}
}
int pop() {
if (isEmpty()) {
printf("Stack is empty\n");
return -1;
} else {
int value = stack[top];
top--;
return value;
}
}
int main() {
push(10);
push(20);
push(30);
printf("%d\n", pop());
printf("%d\n", pop());
printf("%d\n", pop());
printf("%d\n", pop());
return 0;
}
```
以上代码先定义了一个大小为10的数组作为栈,使用top变量来记录栈顶元素的位置。isFull和isEmpty函数分别用来判断栈是否已满和是否为空。push函数将元素压入栈中,如果栈已满则输出提示信息。pop函数将栈顶元素弹出并返回,如果栈空则输出提示信息并返回-1。main函数测试了一下栈的基本操作。
阅读全文
相关推荐
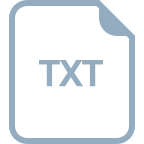
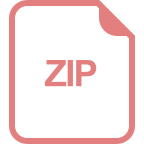
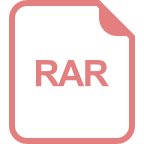
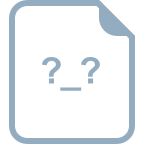