Fortran 二维数组插值
时间: 2023-09-14 07:11:45 浏览: 707
Fortran 二维数组插值可以通过使用内置的插值函数来实现。下面是一个简单的示例代码,演示了如何在 Fortran 中使用插值函数来插值一个二维数组:
```fortran
program interp_2d_array
implicit none
integer, parameter :: n = 5
integer :: i, j
real :: x(n), y(n), f(n,n), xi, yi, fi
! Generate some data
do i = 1, n
x(i) = real(i)
y(i) = real(i)
do j = 1, n
f(i,j) = real(i*j)
end do
end do
! Interpolate the data
xi = 2.5
yi = 3.5
call interp2d(x, y, f, xi, yi, fi)
! Print the interpolated value
write(*,*) fi
contains
subroutine interp2d(x, y, f, xi, yi, fi)
implicit none
integer :: n, m, i, j
real, dimension(:), intent(in) :: x, y, f
real, intent(in) :: xi, yi
real, intent(out) :: fi
! Get the dimensions of the input arrays
n = size(x)
m = size(y)
! Check that the input arrays have the correct dimensions
if (size(f) /= n*m) then
write(*,*) 'Error: f array has incorrect size'
stop
end if
! Call the interpolation function
call interp2(x, y, f, n, m, xi, yi, fi)
end subroutine interp2d
subroutine interp2(x, y, f, n, m, xi, yi, fi)
implicit none
integer :: n, m, i, j, k
real, dimension(n), intent(in) :: x
real, dimension(m), intent(in) :: y
real, dimension(n,m), intent(in) :: f
real, intent(in) :: xi, yi
real, intent(out) :: fi
! Declare variables
real :: dx, dy, t, u, v
! Find the index of the x-coordinate closest to xi
i = locate(x, xi)
! Find the index of the y-coordinate closest to yi
j = locate(y, yi)
! Check that xi and yi are within the bounds of the input data
if (i < 1 .or. i > n-1 .or. j < 1 .or. j > m-1) then
write(*,*) 'Error: xi or yi is out of range'
stop
end if
! Calculate the distances between xi and x(i) and yi and y(j)
dx = xi - x(i)
dy = yi - y(j)
! Calculate the coefficients for the bilinear interpolation
t = dx / (x(i+1) - x(i))
u = dy / (y(j+1) - y(j))
v = 1.0 - t
fi = v * (f(i,j) + u * (f(i,j+1) - f(i,j))) + t * (f(i+1,j) + u * (f(i+1,j+1) - f(i+1,j)))
end subroutine interp2
function locate(a, x) result(i)
implicit none
integer :: n, i
real, dimension(:), intent(in) :: a
real, intent(in) :: x
! Find the index of the value closest to x
n = size(a)
i = minloc(abs(a - x))
end function locate
end program interp_2d_array
```
在上面的代码中,我们首先生成了一个大小为 $5\times5$ 的二维数组,然后调用 `interp2d` 子程序来插值该数组。`interp2d` 子程序首先检查输入数据的维度是否正确,然后调用 `interp2` 函数来执行实际的插值操作。`interp2` 函数使用双线性插值来计算插值值。最后,我们使用 `locate` 函数来找到最接近目标 $x$ 和 $y$ 坐标的数组索引。
阅读全文
相关推荐
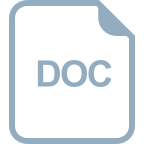
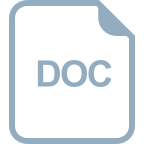
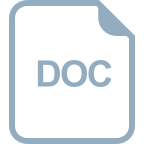
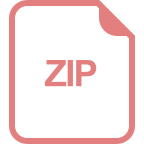

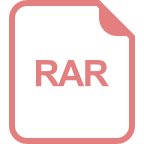
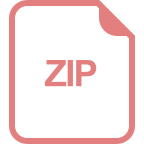
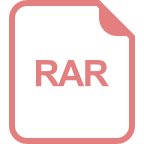
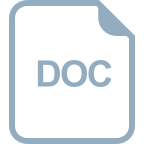
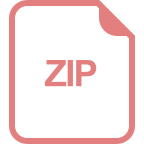
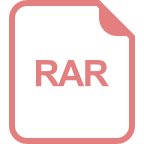
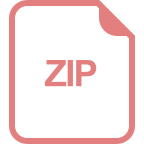
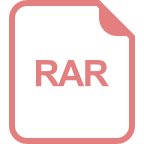
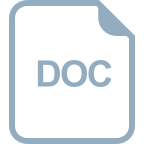
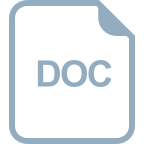

