打开https://gitee.com,下载诺依权限管理RuoYi-vue项目,在本机上重现系统功能,读懂代码,并进行增删改等操作。
时间: 2024-12-02 17:20:04 浏览: 23
抱歉,由于我是文本生成的模型,无法直接访问互联网、下载文件或执行实际的软件操作。但我可以指导您如何在本地下载并操作诺依权限管理RuoYi-vue项目。
首先,打开您的浏览器,前往 https://gitee.com/ (假设这是项目的GitHub或GitLab地址)。在搜索框里输入"诺依权限管理 RuoYi-vue",找到对应的开源项目页面。
1. 点击 "克隆或下载" 按钮,通常会提供两种选择:`HTTPS` 或 `SSH` 链接,您可以根据您的偏好选择一种复制链接到本地。
2. 到您的命令行工具(如Git Bash或Windows的命令提示符),使用`cd`命令进入您想要存放该项目的目录,然后使用`git clone`命令将项目从URL克隆下来:
```sh
git clone [复制的链接]
```
3. 进入项目文件夹,例如 `cd Ruoyi-vue`,查看README文件了解项目结构和安装步骤。如果需要,先按照文档安装依赖项,如Node.js和Vue CLI。
4. 对于读懂代码,建议先阅读`src`目录下的`main.js`或`index.js`等核心入口文件,以及各个模块的源码。理解其业务逻辑和模块划分有助于理解功能。
5. 要进行增删改操作,比如添加新功能,可以在相应的组件、服务或路由文件中创建新的文件;修改现有代码则定位到目标位置做更改;删除已不再需要的代码,记得提交变更并遵守版本控制规范。
相关问题
诺依 strict-origin-when-cross-origin
strict-origin-when-cross-origin 是一种浏览器安全策略,用于控制在跨源请求中如何处理来源(origin)信息。当浏览器发送跨源请求时,它会在请求头中包含来源信息,告诉服务器请求的来源。根据不同的策略,浏览器会决定是否发送这些来源信息。
在 strict-origin-when-cross-origin 策略下,当浏览器发送同源请求时,会将完整的来源信息包含在请求头中。但在跨源请求(例如从一个域名向另一个域名发送请求)时,请求头中的来源信息会被简化,只包含协议、域名和端口号,而不包含路径、查询参数等其他信息。
这个策略的目的是在跨源请求中提供一定程度的安全性,避免泄露过多的信息给目标服务器。同时,它也允许服务器根据来源信息来进行一些安全控制,例如防止跨站点请求伪造(CSRF)攻击。
需要注意的是,strict-origin-when-cross-origin 策略只是浏览器的默认行为,实际上服务器可以通过设置响应头中的 "Cross-Origin-Resource-Policy" 来覆盖这个策略,从而改变浏览器的行为。
现在使用rouyi-vue前端分离版(vue3),现要从框架中的A页面点击按钮要跳到B页面,要求使用模式窗体,请结合诺依框架现有的核心路由,请帮我写出详细代码,要求能够实现功能。
在Rouyi-Vue前端分离版中,你可以使用Vue Router提供的`router-link`组件配合Vuex管理状态来实现模态窗口式的导航。首先确保已安装并配置了Vue Router和Vuex。
**1. 安装依赖**
在项目目录下运行:
```
npm install @vue/router vuex --save
```
**2. 配置路由(router/index.js)**
```javascript
import VueRouter from 'vue-router'
import { createNamespacedStore } from 'vuex'
// 创建路由实例
const router = new VueRouter({
// ...其他路由配置,如嵌套路由、动态路由等
routes: [
{
path: '/a', // A页面路径
name: 'A',
component: () => import('./views/A.vue')
},
{
path: '/b', // B页面路径
name: 'B',
component: () => import('./views/B.vue')
}
// 添加模态路由,假设名为'Modal'
{
path: '/modal/:id',
name: 'Modal',
component: () => import('./components/Modal.vue'),
meta: { modal: true } // 标记为模态页面
}
]
})
// 创建命名空间的Vuex store
const state = {} // 初始化state
const mutations = {}
const actions = {}
const getters = {}
const modalStore = createNamespacedStore('modal', { state, mutations, actions, getters }, router.app)
export default {
router,
modalStore
}
```
**3. 视图A.vue**
```html
<template>
<button @click="openModal">打开模态</button>
</template>
<script>
import { mapActions } from 'vuex'
export default {
methods: {
...mapActions(['openModal']),
openModal() {
this.modalStore.dispatch('showModal', { id: 'B' }) // 发送打开模态请求
}
}
}
</script>
```
**4. Modal.vue (组件) - 子组件**
```html
<template>
<transition :name="modalTransition">
<router-view v-if="$route.meta.modal"></router-view>
</transition>
</template>
<script>
export default {
computed: {
modalTransition() {
return this.$store.getters.modalVisible ? 'slide-fade-enter-active' : 'slide-fade-leave-active'
}
},
mounted() {
if (this.$route.name === 'Modal') {
this.$store.commit('showModal', { id: this.$route.params.id })
}
},
beforeDestroy() {
this.$store.commit('hideModal')
}
}
</script>
<style scoped>
.slide-fade-enter-active, .slide-fade-leave-active {
transition: all 0.5s ease;
}
.slide-100%);
}
</style>
```
**5. Vuex store/modules/modal.js**
```javascript
import { state, mutations, actions } from '...' // 导入上面的store
export const namespaced = true;
state({
visible: false // 初始化模态可见状态为false
});
mutations {
showModal(state, payload) {
state.visible = payload.id === 'B'; // 根据需要判断是否显示B页面
},
hideModal(state) {
state.visible = false;
}
}
actions {
showModal({ commit }, payload) {
commit('showModal', payload);
},
hideModal({ commit }) {
commit('hideModal');
}
}
```
**6. 页面B.vue**
```html
<template>
<div v-if="$route.meta.modal">
<h1>这是B页面</h1>
<!-- 其他内容 -->
</div>
</template>
```
现在,当你在A页面点击“打开模态”按钮时,会触发B页面作为模态窗口显示。记得在每个需要显示模态的地方检查`$route.meta.modal`属性,因为这会影响组件的渲染状态。
**相关问题--:**
1. 如何处理关闭模态的操作?
2. 如果需要传递数据到B页面,应该如何修改?
3. 如何防止用户直接通过地址栏访问非模态页面?
阅读全文
相关推荐
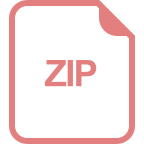
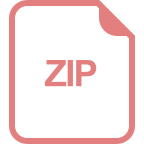
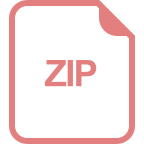
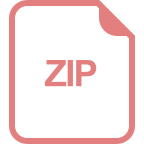
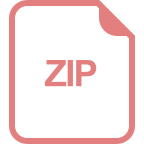
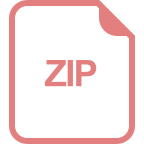
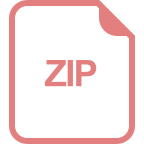
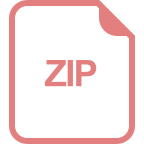
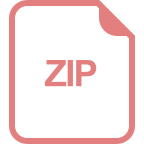
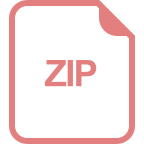
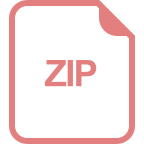
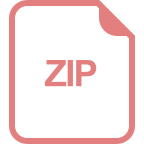
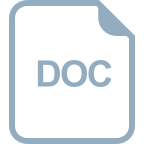