在c++种单向链表的实现
时间: 2024-10-25 14:17:16 浏览: 22
在 C++ 中,单向链表是一种线性数据结构,其中的元素通过指针连接起来,每个节点包含两个部分:数据域用于存储值,以及指向下一个节点的指针域。以下是单向链表的基本实现:
```cpp
// 定义链表节点类 Node
class Node {
public:
int data; // 节点的数据
Node* next; // 指向下一个节点的指针
// 构造函数
Node(int value) : data(value), next(nullptr) {}
};
// 单向链表类 LinkedList
class LinkedList {
private:
Node* head; // 链表头指针
public:
// 初始化链表为空
LinkedList() : head(nullptr) {}
// 插入节点到链表头部
void pushFront(int value) {
Node* newNode = new Node(value);
newNode->next = head;
head = newNode;
}
// 在指定位置插入节点
void insertAfter(int value, int position) {
if (position <= 0)
return;
Node* newNode = new Node(value);
Node* current = head;
for (int i = 0; i < position - 1 && current != nullptr; ++i) {
current = current->next;
}
if (current == nullptr)
return;
newNode->next = current->next;
current->next = newNode;
}
// 删除指定位置的节点
void removeAt(int position) {
if (position <= 0 || head == nullptr)
return;
Node* current = head;
if (position == 1) {
head = head->next;
delete current;
return;
}
for (int i = 1; i < position && current != nullptr; ++i) {
current = current->next;
}
if (current == nullptr)
return;
Node* temp = current->next;
current->next = temp->next;
delete temp;
}
// 打印链表
void printList() {
Node* temp = head;
while (temp != nullptr) {
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
}
// 其他链表操作...
// ...
// 销毁链表(释放内存)
~LinkedList() {
Node* current = head;
Node* next;
while (current != nullptr) {
next = current->next;
delete current;
current = next;
}
}
};
```
阅读全文
相关推荐



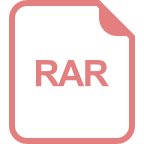











