create a new Pipeline to train a LinearSVC with the following parameters:max_iter: 100000 loss: hinge penalty: l2 (Note: This is 'el-two', and not 'twelve') Use the code cell below to implement your SVM, printing out your training and testing accuraces. Please consult the SciKit Learn documentation on what these parameters mean.
时间: 2024-04-10 09:33:24 浏览: 89
Sure! Here's an example code snippet that creates a pipeline to train a LinearSVC with the specified parameters and prints out the training and testing accuracies:
```python
from sklearn.pipeline import Pipeline
from sklearn.svm import LinearSVC
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from sklearn.preprocessing import StandardScaler
# Load the Iris dataset
data = load_iris()
X = data.data
y = data.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a pipeline with StandardScaler and LinearSVC
pipeline = Pipeline([
('scaler', StandardScaler()),
('svc', LinearSVC(max_iter=100000, loss='hinge', penalty='l2'))
])
# Fit the pipeline on the training data
pipeline.fit(X_train, y_train)
# Predict on the training and testing data
y_train_pred = pipeline.predict(X_train)
y_test_pred = pipeline.predict(X_test)
# Calculate the accuracies
train_accuracy = accuracy_score(y_train, y_train_pred)
test_accuracy = accuracy_score(y_test, y_test_pred)
# Print the accuracies
print("Training Accuracy:", train_accuracy)
print("Testing Accuracy:", test_accuracy)
```
Make sure you have the necessary packages installed (e.g., scikit-learn) and adjust the code according to your specific requirements.
阅读全文
相关推荐
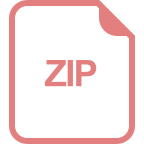
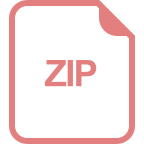
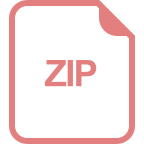
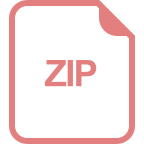
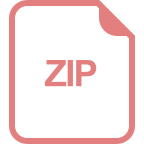
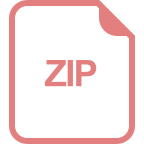
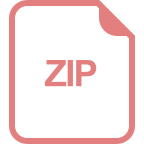
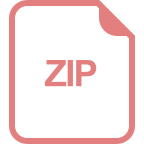
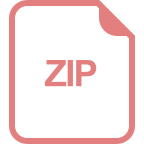
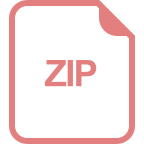
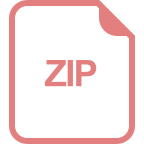
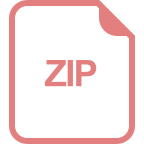
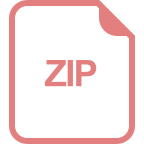
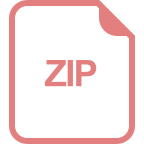
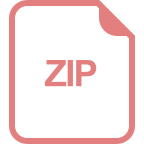
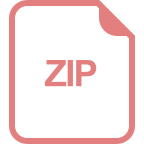
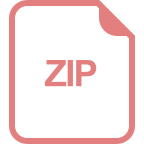
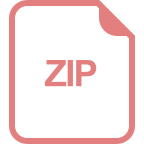
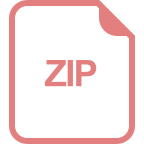