auto hLayout = new QHBoxLayout(); hLayout->addWidget(resultTable()); hLayout->addWidget(resultGelBoxTable());
时间: 2024-04-22 17:26:46 浏览: 212
这段代码使用了 Qt 框架来创建一个水平布局,并向该布局中添加了两个部件。
首先,通过 `auto hLayout = new QHBoxLayout();` 创建了一个 `QHBoxLayout` 对象,并将其赋值给变量 `hLayout`。`auto` 关键字用于自动推断变量的类型,这里推断出的类型是 `QHBoxLayout*`,即指向 `QHBoxLayout` 对象的指针。
接下来,通过 `hLayout->addWidget(resultTable());` 和 `hLayout->addWidget(resultGelBoxTable());` 分别向布局中添加了两个部件。
`resultTable()` 和 `resultGelBoxTable()` 是函数调用,应该返回需要添加到布局中的部件对象。这些函数应该返回一个继承自 `QWidget` 的对象,例如 `QTableWidget` 或者其他自定义的部件类。
这样,通过将这两个部件添加到水平布局中,可以实现这两个部件在水平方向上的排列。最后,可以将该布局应用于父部件,从而显示这些部件的水平布局效果。
相关问题
class MainWindow(QMainWindow): def init(self): super().init() self.setFixedSize(800, 600) main_layout = QVBoxLayout() central_widget = QWidget() central_widget.setLayout(main_layout) self.setCentralWidget(central_widget) button_layout = QVBoxLayout() button1 = QPushButton('当日员工工资') button1.setFixedSize(200, 50) button1.clicked.connect(self.show_query1_result) button_layout.addStretch() button_layout.addWidget(button1) button_layout.addStretch() layout = QHBoxLayout() layout.addStretch() layout.addLayout(button_layout) layout.addStretch() widget = QWidget() widget.setLayout(layout) self.setCentralWidget(widget) main_layout.addLayout(button_layout) self.query1_window = QueryResultWindow() def show_query1_result(self): db = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', db='jj_tset') cursor = db.cursor() db_sql = """ """ cursor.execute(db_sql) result = cursor.fetchall() db.close() if len(result) == 0: QMessageBox.information(self, "提示", "今日无员工工资记录") return self.query1_window.table_widget.setRowCount(0) self.query1_window.table_widget.setColumnCount(len(result[0])) self.query1_window.table_widget.setHorizontalHeaderLabels( ["员工ID", "员工姓名", "日期", "领取鸡爪重量(KG)", "效率(每小时KG)", "出成率", "基础工资", "重量奖励", "当日总工资"]) for row_num, row_data in enumerate(result): self.query1_window.table_widget.insertRow(row_num) for col_num, col_data in enumerate(row_data): self.query1_window.table_widget.setItem(row_num, col_num, QTableWidgetItem(str(col_data))) self.query1_window.show() class QueryResultWindow(QWidget): def init(self): super().init() self.setFixedSize(800, 600) self.table_widget = QTableWidget() self.table_widget.setEditTriggers(QTableWidget.NoEditTriggers) self.table_widget.setSelectionBehavior(QTableWidget.SelectRows) layout = QVBoxLayout() layout.addWidget(self.table_widget) self.setLayout(layout) if name == 'main': app = QApplication(sys.argv) loginWindow = LoginWindow() loginWindow.show() sys.exit(app.exec_()))数据展示页面怎么设置筛选器按ID筛选结果并展示的整体代码
以下是整体代码:
```
import sys
import pymysql
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout, QHBoxLayout, QPushButton, QMessageBox, QTableWidget, QTableWidgetItem
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setFixedSize(800, 600)
main_layout = QVBoxLayout()
central_widget = QWidget()
central_widget.setLayout(main_layout)
self.setCentralWidget(central_widget)
button_layout = QVBoxLayout()
button1 = QPushButton('当日员工工资')
button1.setFixedSize(200, 50)
button1.clicked.connect(self.show_query1_result)
button_layout.addStretch()
button_layout.addWidget(button1)
button_layout.addStretch()
layout = QHBoxLayout()
layout.addStretch()
layout.addLayout(button_layout)
layout.addStretch()
widget = QWidget()
widget.setLayout(layout)
self.setCentralWidget(widget)
main_layout.addLayout(button_layout)
self.query1_window = QueryResultWindow()
def show_query1_result(self):
db = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', db='jj_tset')
cursor = db.cursor()
db_sql = """SELECT * FROM employee_salary WHERE emp_id = %s"""
emp_id, okPressed = QInputDialog.getInt(self, "员工ID筛选", "请输入员工ID:", 0, 0, 999999, 1)
if not okPressed:
return
cursor.execute(db_sql, (emp_id,))
result = cursor.fetchall()
db.close()
if len(result) == 0:
QMessageBox.information(self, "提示", "今日无员工工资记录")
return
self.query1_window.table_widget.setRowCount(0)
self.query1_window.table_widget.setColumnCount(len(result[0]))
self.query1_window.table_widget.setHorizontalHeaderLabels(["员工ID", "员工姓名", "日期", "领取鸡爪重量(KG)", "效率(每小时KG)", "出成率", "基础工资", "重量奖励", "当日总工资"])
for row_num, row_data in enumerate(result):
self.query1_window.table_widget.insertRow(row_num)
for col_num, col_data in enumerate(row_data):
self.query1_window.table_widget.setItem(row_num, col_num, QTableWidgetItem(str(col_data)))
self.query1_window.show()
class QueryResultWindow(QWidget):
def __init__(self):
super().__init__()
self.setFixedSize(800, 600)
self.table_widget = QTableWidget()
self.table_widget.setEditTriggers(QTableWidget.NoEditTriggers)
self.table_widget.setSelectionBehavior(QTableWidget.SelectRows)
layout = QVBoxLayout()
layout.addWidget(self.table_widget)
self.setLayout(layout)
if __name__ == '__main__':
app = QApplication(sys.argv)
mainWindow = MainWindow()
mainWindow.show()
sys.exit(app.exec_())
```
主要是在 `show_query1_result` 方法中添加了一个输入框,用于输入要筛选的员工ID,然后根据输入的ID从数据库中查询数据并展示在表格中。注意要导入 `QInputDialog`,这是一个用于获取用户输入的对话框。
class MainWindow(QMainWindow): def init(self): super().init() # 设置主窗口大小 self.setFixedSize(800, 600) # 创建主窗口布局 main_layout = QVBoxLayout() central_widget = QWidget() central_widget.setLayout(main_layout) self.setCentralWidget(central_widget) # 创建两个竖向按钮 button_layout = QVBoxLayout() button1 = QPushButton('当日员工工资') button1.setFixedSize(200, 50) button1.clicked.connect(self.show_query1_result) button2 = QPushButton('当日鸡爪领取记录') button2.setFixedSize(200, 50) button2.clicked.connect(self.show_query2_result) button3 = QPushButton('查询历史员工工资') button3.setFixedSize(200, 50) button3.clicked.connect(self.show_query3_result) button4 = QPushButton('查询历史鸡爪领取记录') button4.setFixedSize(200, 50) button4.clicked.connect(self.show_query4_result) button_layout.addStretch() button_layout.addWidget(button1) button_layout.addWidget(button2) button_layout.addWidget(button3) button_layout.addWidget(button4) button_layout.addStretch() layout = QHBoxLayout() layout.addStretch() layout.addLayout(button_layout) layout.addStretch() widget = QWidget() widget.setLayout(layout) self.setCentralWidget(widget) # 将按钮布局添加到主窗口布局中 main_layout.addLayout(button_layout) # 创建两个窗口用于展示查询结果 self.query1_window = QueryResultWindow() self.query2_window = QueryResultWindow() self.query3_window = QueryResultWindow() self.query4_window = QueryResultWindow() def show_query1_result(self): # 查询数据 db = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', db='jj_tset') cursor = db.cursor() db_sql = """ """ cursor.execute(db_sql) result = cursor.fetchall() db.close() if len(result) == 0: QMessageBox.information(self, "提示", "今日无员工工资记录") return # 清空表格 self.query1_window.table_widget.setRowCount(0) self.query1_window.table_widget.setColumnCount(len(result[0])) self.query1_window.table_widget.setHorizontalHeaderLabels \ (["员工ID", "员工姓名", "日期", "领取鸡爪重量(KG)", "效率(每小时KG)", "出成率", "基础工资", "重量奖励", "当日总工资"]) # 添加数据到表格 for row_num, row_data in enumerate(result): self.query1_window.table_widget.insertRow(row_num) for col_num, col_data in enumerate(row_data): self.query1_window.table_widget.setItem(row_num, col_num, QTableWidgetItem(str(col_data))) # 显示窗口 self.query1_window.show()数据展示页面怎么设置筛选器按ID筛选结果并展示
你可以在数据展示页面添加一个 QLineEdit 和一个 QPushButton,让用户输入员工ID并点击按钮进行筛选。具体实现可以按照以下步骤进行:
1. 在数据展示页面的构造函数中创建 QLineEdit 和 QPushButton,并添加到布局中。
```python
self.filter_edit = QLineEdit()
self.filter_button = QPushButton('筛选')
self.filter_button.clicked.connect(self.filter_result)
button_layout.addWidget(self.filter_edit)
button_layout.addWidget(self.filter_button)
```
2. 创建一个方法 `filter_result` 用于筛选结果。该方法首先获取用户输入的员工ID,然后查询数据库获取符合条件的记录,最后将结果添加到表格中。
```python
def filter_result(self):
employee_id = self.filter_edit.text()
if not employee_id:
return
db = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', db='jj_tset')
cursor = db.cursor()
db_sql = f"SELECT * FROM employee_salary WHERE employee_id={employee_id}"
cursor.execute(db_sql)
result = cursor.fetchall()
db.close()
if len(result) == 0:
QMessageBox.information(self, "提示", "无符合条件的记录")
return
self.table_widget.setRowCount(0)
for row_num, row_data in enumerate(result):
self.table_widget.insertRow(row_num)
for col_num, col_data in enumerate(row_data):
self.table_widget.setItem(row_num, col_num, QTableWidgetItem(str(col_data)))
```
3. 将 QLineEdit 和 QPushButton 添加到布局中,并显示窗口即可。
完整代码如下:
```python
class QueryResultWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('查询结果')
self.setFixedSize(800, 600)
main_layout = QVBoxLayout()
central_widget = QWidget()
central_widget.setLayout(main_layout)
self.setCentralWidget(central_widget)
table_widget = QTableWidget()
table_widget.setColumnCount(9)
table_widget.setHorizontalHeaderLabels(["员工ID", "员工姓名", "日期", "领取鸡爪重量(KG)", "效率(每小时KG)", "出成率", "基础工资", "重量奖励", "当日总工资"])
main_layout.addWidget(table_widget)
button_layout = QHBoxLayout()
self.filter_edit = QLineEdit()
self.filter_button = QPushButton('筛选')
self.filter_button.clicked.connect(self.filter_result)
button_layout.addWidget(self.filter_edit)
button_layout.addWidget(self.filter_button)
button_layout.addStretch()
main_layout.addLayout(button_layout)
self.table_widget = table_widget
def filter_result(self):
employee_id = self.filter_edit.text()
if not employee_id:
return
db = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', db='jj_tset')
cursor = db.cursor()
db_sql = f"SELECT * FROM employee_salary WHERE employee_id={employee_id}"
cursor.execute(db_sql)
result = cursor.fetchall()
db.close()
if len(result) == 0:
QMessageBox.information(self, "提示", "无符合条件的记录")
return
self.table_widget.setRowCount(0)
for row_num, row_data in enumerate(result):
self.table_widget.insertRow(row_num)
for col_num, col_data in enumerate(row_data):
self.table_widget.setItem(row_num, col_num, QTableWidgetItem(str(col_data)))
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐
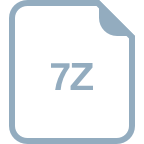
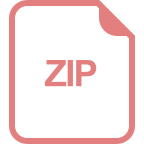
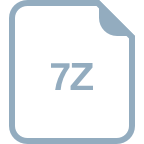
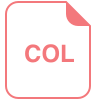





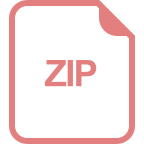
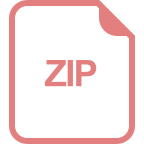