输入一个isbn 13码,首先通过调解算法判断是否合法。试着输出对应的sb n时编码中间没有空格,否则输出error。
时间: 2024-11-15 16:31:34 浏览: 4
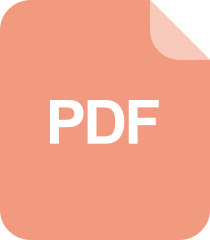
基于案例推理的社区纠纷调解方案检索算法
ISBN (International Standard Book Number) 13码是一种用于书籍、期刊和其他出版物的身份标识符,由13个数字组成。要验证一个ISBN-13码并检查其中是否有空格,你可以使用以下步骤:
1. **长度验证**:
- 首先确保给定字符串至少包含13个字符。
2. **校验位**:
- ISBN-13码的最后一位(校验位)是通过特定算法计算得出的,基于前12位的奇偶性。你可以用模10算法(X * 3 + Y, X为奇数位乘3的和,Y为偶数位的和,然后对10取余)来确定校验位。
3. **格式检查**:
- 检查码中的每个数字是否都是数字字符(0-9)。如果有非数字字符,如空格,则输出`error`。
4. **去除空格**:
- 如果发现有空格,删除它们并将字符串恢复到原始状态进行后续处理。
以下是一个简单的C++代码示例,实现了这些功能:
```cpp
#include <iostream>
#include <string>
bool isValidISBN13(const std::string& isbn13) {
if (isbn13.length() != 13 || isbn13.find_first_not_of("0123456789") != std::string::npos) {
return false;
}
int sum = 0;
bool alternate_multiplication = false;
for (char digit : isbn13) {
digit -= '0';
if (alternate_multiplication) {
sum += digit * 3;
} else {
sum += digit;
}
alternate_multiplication = !alternate_multiplication;
}
// 校验位计算
int remainder = sum % 10;
if (remainder == 0) {
return true; // Even number of digits, so last digit is a 0
} else {
return (10 - remainder) % 10 == isbn13.back() - '0'; // Odd number of digits, compare with calculated check digit
}
}
std::string formatWithoutSpaces(const std::string& isbn13) {
if (!isValidISBN13(isbn13)) {
return "error";
}
return isbn13.replace(isbn13.find(' '), 1, "");
}
int main() {
std::string isbn;
std::cout << "Enter an ISBN-13 code without spaces: ";
std::cin >> isbn;
std::string formattedIsbn = formatWithoutSpaces(isbn);
if ("error" == formattedIsbn) {
std::cout << "Invalid ISBN-13.\n";
} else {
std::cout << "Formatted ISBN-13: " << formattedIsbn << "\n";
}
return 0;
}
```
阅读全文
相关推荐
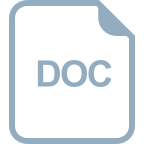
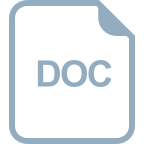
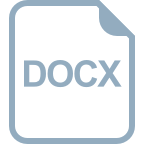
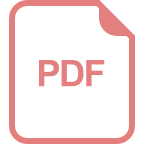
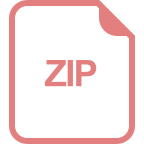
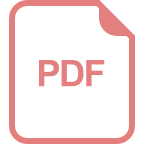
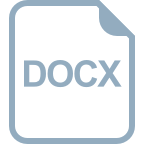
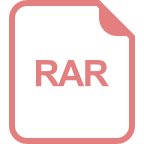
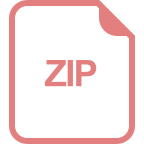
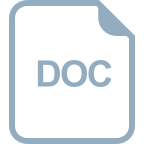
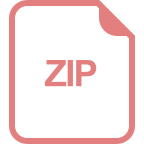
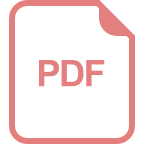
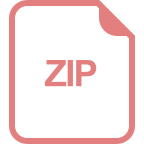
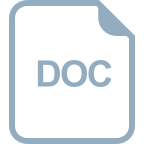
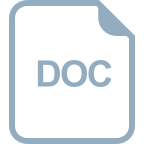