完整的3d旋转相册带背景和音乐的代码
时间: 2024-09-28 15:17:26 浏览: 66
创建一个完整的3D旋转相册,包括背景和音乐功能,需要涉及到多个库和一些复杂的编程技巧。这通常是在WebGL、JavaScript(如Three.js库)和HTML5的环境中完成的。以下是一个简化的概述:
```javascript
// 引入Three.js库和其他必要的依赖
import * as THREE from 'three';
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls';
// 初始化场景、相机和渲染器
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer({ antialias: true });
renderer.setSize(window.innerWidth, window.innerHeight);
// 添加背景
const backgroundGeo = new THREE.PlaneGeometry(1000, 1000);
const backgroundMat = new THREE.MeshBasicMaterial({ color: 0x808080 }); // 灰色背景
const backgroundMesh = new THREE.Mesh(backgroundGeo, backgroundMat);
scene.add(backgroundMesh);
// 创建3D图片数组并添加到场景
let images = ...; // 图片数据,包含URL和3D位置信息
images.forEach((image) => {
const imgLoader = new THREE.TextureLoader();
imgLoader.load(image.url, (texture) => {
const cubeGeo = new THREE.BoxGeometry(200, 200, 200); // 相册立方体大小
const mesh = new THREE.Mesh(cubeGeo, new THREE.MeshBasicMaterial({ map: texture }));
mesh.position.set(image.x, image.y, image.z); // 设置3D位置
scene.add(mesh);
});
});
// 控制器
const controls = new OrbitControls(camera, renderer.domElement);
controls.update();
// 渲染函数
function animate() {
requestAnimationFrame(animate);
controls.update();
renderer.render(scene, camera);
}
// 音乐加载和播放
const audioLoader = new THREE.AudioLoader();
audioLoader.load('music.mp3', (audio) => {
audio.play(); // 开始播放音乐
});
animate();
//
阅读全文
相关推荐
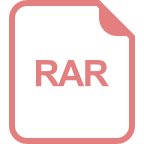
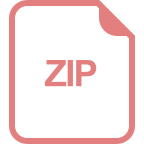
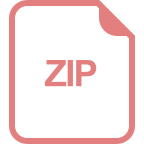
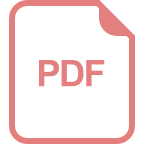
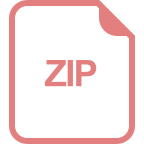
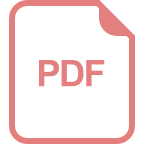
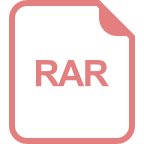
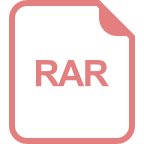
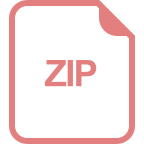
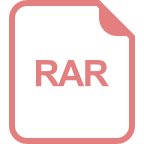
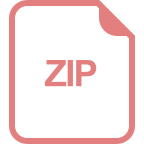
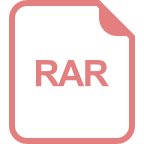
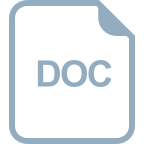
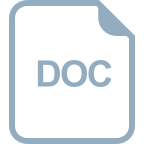
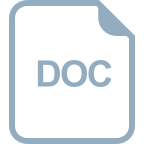
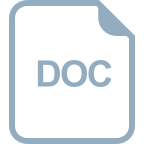
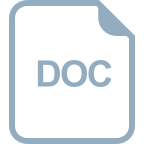

