1.编写一个Java应用程序,该程序中有两个类:Vehicle(用于刻画机动车)和User(主类)。
时间: 2024-11-09 20:30:53 浏览: 37
在Java中,你可以创建一个简单的应用程序来展示多态的概念。首先,我们定义一个名为`Vehicle`的抽象类,它代表所有车辆的基本特性:
```java
abstract class Vehicle {
private String type;
public Vehicle(String type) {
this.type = type;
}
// 定义抽象方法,每个具体的车辆类型需要实现这个方法
abstract void drive();
public String getType() {
return type;
}
}
```
接着,我们创建两个具体的子类,比如`Car`和`Motorcycle`,它们继承自`Vehicle`并重写`drive()`方法:
```java
class Car extends Vehicle {
public Car(String type) {
super(type);
}
@Override
public void drive() {
System.out.println(getType() + " is driving on the road.");
}
}
class Motorcycle extends Vehicle {
public Motorcycle(String type) {
super(type);
}
@Override
public void drive() {
System.out.println(getType() + " is riding on the highway.");
}
}
```
然后,在`User`类中,我们可以创建一个方法来处理不同类型车辆的操作,利用多态性:
```java
public class User {
public void operate(Vehicle vehicle) {
vehicle.drive();
}
}
```
在`main`函数里,用户可以实例化不同类型的车辆并操作它们:
```java
public static void main(String[] args) {
User user = new User();
Vehicle car = new Car("Toyota");
Vehicle motorcycle = new Motorcycle("Harley-Davidson");
user.operate(car); // 输出: Toyota is driving on the road.
user.operate(motorcycle); // 输出: Harley-Davidson is riding on the highway.
}
```
在这个例子中,`operate`方法接受一个`Vehicle`类型的参数,但实际上可以根据传入的具体子类实例执行相应的`drive`方法,这就是多态的体现。
阅读全文
相关推荐
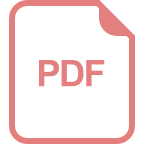
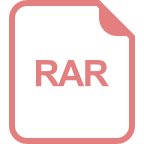
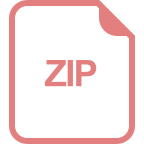








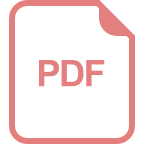

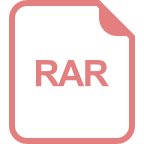
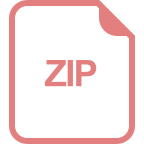
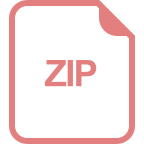
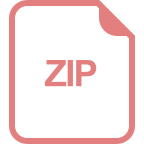
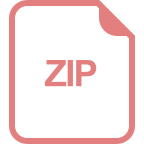