java简单的五子棋
时间: 2023-11-24 07:50:25 浏览: 86
以下是一个简单的Java五子棋游戏的实现,其中包括棋盘的绘制和落子的判断:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Gobang extends JFrame implements Config {
private int[][] board = new int[COUNT][COUNT]; // 棋盘数组,0表示空,1表示黑子,2表示白子
private int player = 1; // 当前玩家,1表示黑方,2表示白方
public Gobang() {
setTitle("五子棋");
setSize(600, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setResizable(false);
addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
int x = e.getX();
int y = e.getY();
int i = (x - X0 + SIZE / 2) / SIZE;
int j = (y - Y0 + SIZE / 2) / SIZE;
if (i >= 0 && i < COUNT && j >= 0 && j < COUNT && board[i][j] == 0) {
board[i][j] = player;
repaint();
if (checkWin(i, j)) {
JOptionPane.showMessageDialog(null, (player == 1 ? "黑方" : "白方") + "获胜!");
reset();
} else {
player = 3 - player;
}
}
}
});
setVisible(true);
}
public void paint(Graphics g) {
super.paint(g);
for (int i = 0; i < COUNT; i++) {
g.drawLine(X0, Y0 + i * SIZE, X0 + (COUNT - 1) * SIZE, Y0 + i * SIZE);
g.drawLine(X0 + i * SIZE, Y0, X0 + i * SIZE, Y0 + (COUNT - 1) * SIZE);
}
for (int i = 0; i < COUNT; i++) {
for (int j = 0; j < COUNT; j++) {
if (board[i][j] == 1) {
g.setColor(Color.BLACK);
g.fillOval(X0 + i * SIZE - SIZE / 2, Y0 + j * SIZE - SIZE / 2, SIZE, SIZE);
} else if (board[i][j] == 2) {
g.setColor(Color.WHITE);
g.fillOval(X0 + i * SIZE - SIZE / 2, Y0 + j * SIZE - SIZE / 2, SIZE, SIZE);
}
}
}
}
private boolean checkWin(int i, int j) {
int count = 1;
for (int k = 1; k < 5 && i + k < COUNT && board[i + k][j] == player; k++) count++;
for (int k = 1; k < 5 && i - k >= 0 && board[i - k][j] == player; k++) count++;
if (count >= 5) return true;
count = 1;
for (int k = 1; k < 5 && j + k < COUNT && board[i][j + k] == player; k++) count++;
for (int k = 1; k < 5 && j - k >= 0 && board[i][j - k] == player; k++) count++;
if (count >= 5) return true;
count = 1;
for (int k = 1; k < 5 && i + k < COUNT && j + k < COUNT && board[i + k][j + k] == player; k++) count++;
for (int k = 1; k < 5 && i - k >= 0 && j - k >= 0 && board[i - k][j - k] == player; k++) count++;
if (count >= 5) return true;
count = 1;
for (int k = 1; k < 5 && i + k < COUNT && j - k >= 0 && board[i + k][j - k] == player; k++) count++;
for (int k = 1; k < 5 && i - k >= 0 && j + k < COUNT && board[i - k][j + k] == player; k++) count++;
if (count >= 5) return true;
return false;
}
private void reset() {
for (int i = 0; i < COUNT; i++) {
for (int j = 0; j < COUNT; j++) {
board[i][j] = 0;
}
}
player = 1;
repaint();
}
public static void main(String[] args) {
new Gobang();
}
}
interface Config {
public static final int X0 = 25;
public static final int Y0 = 25;
public static final int SIZE = 35;
public static final int COUNT = 15;
}
```
阅读全文
相关推荐
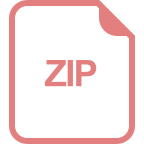
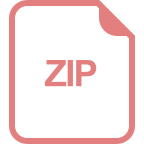
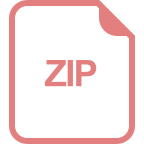
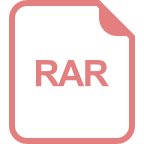
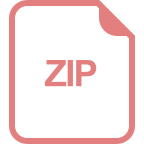
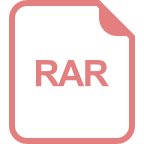
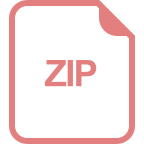
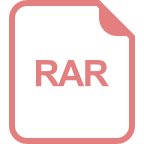
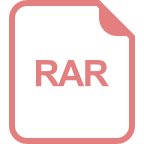
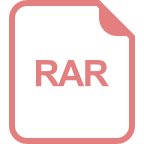
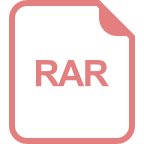
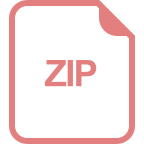
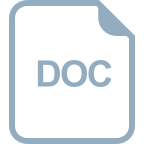
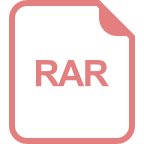
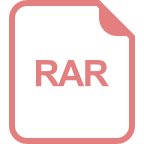
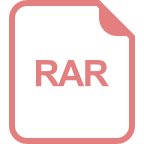